ASP.NET websites do not allow you to use multiple programming languages such as C# and Visual Basic in the App_Code folder of the website project. If you are creating a C# web site and you have copied a Visual Basic source file in App_Code folder, you won’t be able to compile your ASP.NET website. Off course, if it is a small source file, then you can convert it into C# source code either yourself or by using any code converter but it is time wasting practice if you have a large file or you want to use multiple source files. In this tutorial, I will show you how you can use multiple programming languages source files in a single website project by just doing some simple modifications in the configuration settings of the website.
To get things started, create an ASP.NET website project using either Visual Basic or C# in Visual Studio. I am creating a C# website project for this tutorial. Now right click the website project name in Solution Explorer and add the Add App_Code folder in the website from the “Add ASP.NET Folder” menu option. Next you need to create a separate subfolder for each of the programming language you want to support in your website. For the purpose of this tutorial I am adding CS and VB subfolders to support C# and Visual Basic source files. For this tutorial I am creating two simple code files named “ClassOne.cs” and “ClassTwo.vb” in CS and VB subfolders respectively. Your website structure should look like the following figure after the files are created.
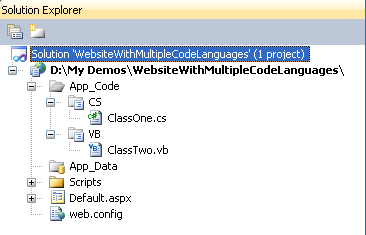
To check whether these files compile and work properly, I am adding a field and a function in both files. The source code of both files is shown below:
ClassOne.cs
public class ClassOne
{
public string Message;
public string GetMessage()
{
return this.Message;
}
}
ClassTwo.vb
Public Class ClassTwo
Public Message As String
Public Function GetMessage() As String
Return Me.Message
End Function
End Class
After creating separate subfolders for each programming language and creating multiple source files, all you need is simple modification in your website web.config file to tell ASP.NET to compile the files located in those subfolders separately. Open the web.config file of the website project and locate <compilation> the section in the file. You need to add the following <codesubdirectories> section inside the <compilation> section.
<compilation>
<codeSubDirectories>
<add directoryName="CS"/>
<add directoryName="VB"/>
</codeSubDirectories>
</compilation>
That’s all is required to support multiple languages in ASP.NET website project. You can now use both classes as normally in your website. To test these classes, create a Default.aspx page if it is not already created in your project. Add Two buttons and a label control on it and you can use those two classes on the button click events of both buttons as shown in the code below:
protected void Button1_Click(object sender, EventArgs e)
{
ClassOne obj = new ClassOne();
obj.Message = "C# Function is called...";
Label2.Text = obj.GetMessage();
}
protected void Button2_Click(object sender, EventArgs e)
{
ClassTwo obj = new ClassTwo();
obj.Message = "VB Function is called...";
Label2.Text = obj.GetMessage();
}
Following is the output of the page.
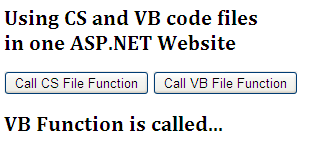
Developers commonly include multiple programming languages in Web applications to support multiple development teams that operate independently and prefer different programming languages. I hope I am able to teach you how you can support multiple programming languages in ASP.NET website project.
I do follow what you are saying in the post of information for application development. expecting more updated information.
nice and useful information
Great Article
Sir,
Love to read your every article..
very nice article
So simply you explained. Nice tutorial
thankx such a useful information
.