GridView control is one of the most powerful controls in ASP.NET 2.0. This control displays database records in typical tabular form, and provides features such as Sorting, Paging, Selection and Editing without writing a single line of code. It also has many different types of fields (columns) such as hyperlinks, images, checkboxes etc.
In the following tutorial, I will show you different techniques you can use to display command buttons in GridView. For this tutorial I am using Microsoft famous sample database Northwind. I am displaying Products table in the GridView. Also make sure you are setting GridView DataKeyFields property to the Primary Key column of the Product Table such as ProductID. GridView control RowCommand event will give you the ProductID of the product from the GridView Rows when user will click any of the button in the GridView.
Here is the code of GridView control.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false"
BorderColor="#336699" BorderStyle="Solid" BorderWidth="2px"
CellPadding="4" DataKeyNames="ProductID"
DataSourceID="SqlDataSource1" ForeColor="#333333">
<Columns>
<asp:BoundField DataField="ProductID" HeaderText="ProductID"
SortExpression="ProductID" />
<asp:ButtonField ButtonType="link" CommandName="ProductName"
DataTextField="ProductName" HeaderText="Name"
SortExpression="ProductName" />
<asp:ButtonField ButtonType="button" CommandName="MoreDetail"
HeaderText="More Details" Text="More Details" />
<asp:ButtonField ButtonType="Link" CommandName="ViewCategory"
HeaderText="View Category" Text="View Category" />
<asp:ButtonField ButtonType="Image" CommandName="BuyNow"
HeaderText="Buy Now" ImageUrl="buynow.gif" />
<asp:ButtonField DataTextField="UnitsInStock" HeaderText="Stock"
ButtonType="button" DataTextFormatString="{0} Items"
CommandName="Stock" />
</Columns>
</asp:GridView>
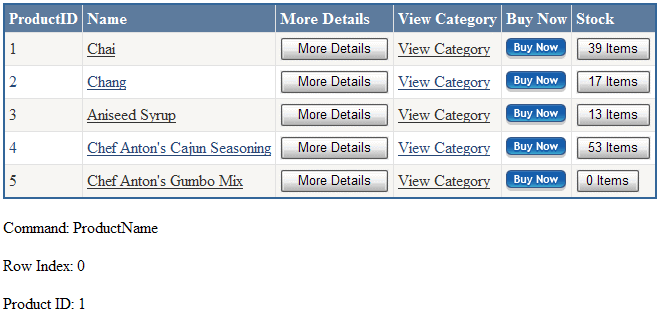
To handle click event of the buttons in GridView you need to handle RowCommand event of the GridView control. Following code will show you how you can get RowIndex, ProductID and CommandName of the button when user click any button in the GridView.
VB.NET
Protected Sub GridView1_RowCommand(ByVal sender As Object,
ByVal e As System.Web.UI.WebControls.GridViewCommandEventArgs)
Handles GridView1.RowCommand
Dim currentCommand As String = e.CommandName
Dim currentRowIndex As Integer = Int32.Parse(e.CommandArgument.ToString())
Dim ProductID As String = GridView1.DataKeys(currentRowIndex).Value
Label1.Text = "Command: " & currentCommand
Label2.Text = "Row Index: " & currentRowIndex.ToString
Label3.Text = "Product ID: " & ProductID
End Sub
C#
protected void GridView1_RowCommand(object sender,
System.Web.UI.WebControls.GridViewCommandEventArgs e)
{
string currentCommand = e.CommandName;
int currentRowIndex = Int32.Parse(e.CommandArgument.ToString());
string ProductID = GridView1.DataKeys[currentRowIndex].Value;
Label1.Text = "Command: " + currentCommand;
Label2.Text = "Row Index: " + currentRowIndex.ToString;
Label3.Text = "Product ID: " + ProductID;
}
for information. Can add a OnRowCommand on asp:gridview link with GridView1_RowCommand is work on VS2012.
Thx admin giving this info.
Thanks a lot! Very useful.
Martin
Nice..very useful…..is t possible to update the values of particular cell in a grid by a butoon click ??????
ex: gridview1.rows[2].cells[2] value sholud be decrementd for every button click ….
many thanks, works perfect, best code ever.
Thanks :)) this was realy Simple & useful
Unbelivable, spent two days trying to figure this out. Thank you very much! You have solved my 2012 .NET 4 problem with a solution from 2008 .NET 2. 🙂
Thank you so much! This has been extremely helpful! 🙂
Thanks, this helped me 🙂
Thanks……………
Informaticz
Software Company Thrissur
thanks dude,you got simple tutorial and its very good.:D
Thanks a lot. This page helped me a lot to complete my project.
I see how to get a grid view to display the buttons in a column. Now how do you get the those buttons to display data when clicked?
I am trying to fill a 2nd grid view using input parameters including data from the 1st grid view rows using a stored procedure when the button in that row is clicked. Please help.
Hi david menke
In this tutorial, you can see how to do that using RowCommand event. Once you get product id you can get records related to that product id and display records on second grid.
very helpful tutorial, thanks for the tutorial.
Sir Thank You Very Much for great tutorial
what is lablel1, label2, label3, please help…
Label1, Label2 and Label3 are simple ASP.NET Label controls.
this is really a good site
Clean, Precise and good code.
thanks! it was extremely useful
Thank u
Thanks. It was very helpful. After correcting syntax errors, it worked perfectly fine. Thanks again.
what about delete event?
Presented simply and works!!
Nice, thanks. You would not believe how many over-complicated solutions to this problem show up in Google before you get to this perfectly straightforward one!
By the way, im using image button to select the id. Is there a way to click on the imagebutton to retreive the same result as yours without me selecting ont the ‘select’ button?
Hi it works only for my first page, as there are many pages in my gridview so when i click on the others pages it show:
Index was out of range. Must be non-negative and less than the size of the collection.
Parameter name: index
Please help.
thanks a lot – you have helped me fix a problem that was buggin me!
Excelent, symple and easy
Thanks boss it was very useful
Everything is perfect except for the usage of ( ) insted of [ ] for the rowindex
The code did not work.. It had some syntax mistakes(The c# one).
I fixed the syntax mistakes and it didnt work still.
The most important thing for me is to get the index of the row on which the button was clicked.
please try to reply.
Hi
You make sure that DataKeyFields property is set as the primary key column of your datasource.