Web Services play an important role in data transport and communication between the client the server. Web services reside on the server and wait to be called by any client and most of the time these clients are written in server side technologies such as ASP.NET, PHP or JSP etc. ASP.NET AJAX provides web developers ability to call web services from the client side language such as JavaScript asynchronously to improve the user experience and to avoid full page refresh and postback. In this tutorial, I will show you how you can call an ASP.NET web service with the help of ASP.NET AJAX.
For the purpose of this tutorial I have created a web service with the name ProductsService.asmx. If you are new to web services and don’t know how to add web service right click on your website name in Solution Explorer and click Add New Item option and select the Web Service icon from the available file templates as shown in figure below. A new file with the name ProductsService.asmx will be added in your website and the code behind file for the web service will be added in App_Code folder.
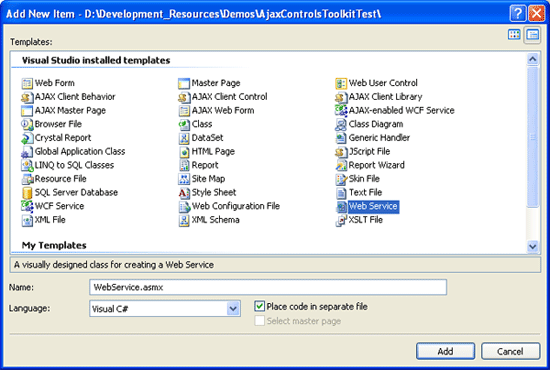
Notice the ProductsService class is derived from the System.Web.Services.WebService class available in .NET Framework class library. Also check the following directive in your code and make sure it is not commented because this directive is required to call web service using ASP.NET AJAX.
[System.Web.Script.Services.ScriptService]
The implementation of web service is fairly straight forward. It has only one method GetProducts which is decorated with [WebMethod] attribute that is required to expose web service methods to consumers and calling applications. The method takes category id as a parameter and return some products based on the category. I am returning hard coded products names and not connecting database in this tutorial but you can easily write code to return products from the database.
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.Web.Script.Services.ScriptService]
public class ProductsService : System.Web.Services.WebService
{
[WebMethod]
public List<string> GetProducts(int categoryId)
{
List<string> list = new List<string>();
if (categoryId == 1)
{
list.Add("Nokia N95");
list.Add("Nokia N70");
list.Add("Nokia 6630");
}
else
{
list.Add("Dell Laptop");
list.Add("HP Laptop");
list.Add("Sony Laptop");
}
return list;
}
}
To consume web service by using client script you need to add an instance of the ASP.NET AJAX ScriptManager control in your page. This control supports a Services collection which can be used to add the reference of web services you want to call from client side JavaScript. The ASP.NET AJAX asynchronous communication layer automatically generates JavaScript proxy classes for each Web service reference you add using ScriptManager control.
Once your page is requested, the proxy class is downloaded to the browser at the page load time and provides a client object that represents the exposed methods of a Web service. To call a method of the Web service, you can call corresponding method of generated proxy class. The proxy class in turn communicates with the Web service asynchronously by using XmlHttpObject of the browser.
For this tutorial, I have added the reference of ProductsService.asmx by using <asp:ScriptReference> element inside Services collection under the <asp:ScriptManager> element. The remaining form has a DropDownList, an HTML button and a Label control. Notice that the onclick event of the Button1 control is calling a JavaScript function Button1_onclick() which needs to be defined in the JavaScript in your page.
<form id="form1" runat="server">
<asp:ScriptManager ID="ScriptManager1" runat="server">
<Services>
<asp:ServiceReference Path="~/ProductsService.asmx" />
</Services>
</asp:ScriptManager>
Select Category:
<asp:DropDownList ID="DropDownList1" runat="server">
<asp:ListItem Value="1">Mobiles</asp:ListItem>
<asp:ListItem Value="2">Laptops</asp:ListItem>
</asp:DropDownList>
<input id="Button1" type="button" value="Get Products"
onclick="Button1_onclick()" />
<br />
<br />
<asp:Label ID="Label1" runat="server"></asp:Label>
</form>
As I mentioned earlier that calling a Web service method from client script is asynchronous means the communication will take place behind the scene and user will remain interactive with the page. If your web service returns some data you must provide a callback function you want to execute on successful call to web service. You can also provide another callback function to handle errors in case the Web service call failed due to any communication or server error or Web service return no data.
I have added the following JavaScript code in my page to call Web service. Notice how I have called the GetProducts method of the Web service using the ProductsService proxy class generated automatically for me when I have added the Web service reference using ScriptManager control. Also notice the callback functions onSuccess and onFailed have been passed as a second and third parameter in the proxy class GetProducts method to receive the results or error messages returned by the Web service and to update the page accordingly.
<head runat="server">
<title>Consuming Web Services From AJAX</title>
<script type="text/javascript">
function Button1_onclick()
{
var categoryId = $get("DropDownList1").value;
ProductsService.GetProducts(categoryId, onSuccess, onFailed);
}
function onSuccess(result)
{
var Label1 = $get("Label1");
Label1.innerHTML = "";
for(i=0 ; i<result.length ; i++)
{
Label1.innerHTML += result[i] + "<br />";
}
}
function onFailed(error)
{
var Label1 = $get("Label1");
Label1.innerHTML = "Service Error: " + error.get_message();
}
</script>
</head>
Build your project and test your page in the browser and you will see output similar to the following when you will click the button after selecting a category from the DropDownList.
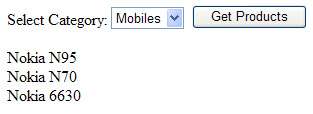
nice article dear
Nice article. How we can serialize Entity Framework Objects to JSON? It will be very helpful for us if you write an article on this topic.
web service is not getting called , when you are adding it to the app_code folder in website. (web application)
Hi,
Nice article, but we can do the same call to webservice by putting controls in the update panel and write a server side function on button click.
actually i m trying to undrstand in what scenerios this technique is useful more.
Sir how can i consume the free services that u have exposed at ur site. Plz give an article on that too as it will be quite useful as some sites have exposed some free services for sending SMS to mobiles hence ur article in this regard will be more helpful
Regards
nice article sir
thanks!!!!