Saving and displaying images in database tables is a common requirement in ASP.NET projects. There are two ways to store images in database either you store image URLs in database as normal string or you store image as binary data. In this tutorial I will show you how you can upload and save images directly in SQL Server database table as binary data.
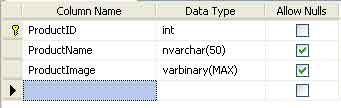
Now let’s take a look at the form I am going to use, to upload images to the above table.
<form id="form1" runat="server">
Product Name:
<asp:TextBox id="txtProductName" runat="server" />
<br />
Product Image:
<asp:FileUpload id="FileUpload1" runat="server" />
<asp:Button id="btnSave" runat="server" Text="Save Product" onclick="btnSave_Click" />
<br /><br />
<asp:Label id="lblMessage" runat="server" />
</form>
The above code looks pretty straightforward as it is just showing one TextBox, one ASP.NET 2.0 FileUpload control and a Button to Save Product Name and Image in database as shown in the following figure:
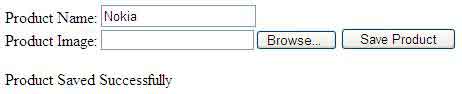
Following is the C# code for Save Product button click event.
protected void btnSave_Click(object sender, EventArgs e)
{
if(FileUpload1.HasFile)
{
string productName = txtProductName.Text;
byte[] productImage = FileUpload1.FileBytes;
string constr = "Server=TestServer; Database=SampleDB; uid=sa; pwd=abc123";
string query = "INSERT INTO Products(ProductName, ProductImage) VALUES(@ProductName, @ProductImage)";
SqlConnection con = new SqlConnection(constr);
SqlCommand com = new SqlCommand(query, con);
com.Parameters.Add("@ProductName", SqlDbType.NVarChar).Value = productName;
com.Parameters.Add("@ProductImage", SqlDbType.VarBinary).Value = productImage;
con.Open();
int result = com.ExecuteNonQuery();
con.Close();
if (result > 0)
{
lblMessage.Text = "Product Saved Successfully";
}
}
else
{
lblMessage.Text = "Please Select Product Image File";
}
}
The above code is running simple SQL Insert query to store images in database table. FileUpload control HasFile property checks whether user has selected a file or not. FileBytes property returns the image file as the array of bytes.
If you want to upload the image file to a File System then you can also use FileUpload SaveAs method that requires the server path to upload the file. If you want to verify the file size and its type then you can use FileUpload.PostedFile.ContentLength and FileUpload.PostedFile.ContentType properties.
THANKS A LOT, AND GOD BLESS YOU
THANKS A LOT, AND GOD BLESS YOU
I search on internet for it
and I very confused with a lot of complex code
I lost my hope
I obtain fantastic feel with your awesome help
Hi, Great job,
Wow Man Amazing Simple and Brilliant
Wow Man Amazing Simple and Brilliant
thanks alot sir for helping me to my programe.
Hello,Sir I want to save multiple values database so how can i save.
Hello,Sir I want to save multiple values database so how can i save.
Great…
Its works at first try….
Awesome…..
Great…
Its works at first try….
Awesome…..
Thanks a lot! Great work!
very nice , easy and clear solution,..
very nice , easy and clear solution,..
First thanks for your post.. But when i saved an image and opened the database it shows 0px. what does it mean.is the image saved successfully or not…???? Plz leave a reply to my mail.
Its goooooood
Its goooooood
Thanks a lot.
Could you please send me this Saving Image In Database in ASP.NET 4 VB Code.
Thanks in Advance,
Mawsar
Nice article……. easy to understand…..
thanks….
This is really helpful.
What i did with my example i am storing files but not images,
My question is how do i retrive this files from the database?
otherwise storing files its working.
Thanks alot
Sir,Please tell me about sqldbtype,Hasfile,File bytes
Sir,Please tell me about sqldbtype,Hasfile,File bytes
after uploading how to display image in listview from varbinary datatype?
Thanks for posting this code, very helpful
Thanks for posting this code, very helpful
Thanxxxx…but how can I store image URLs in database as normal string ….Plzzzz Help…..
Thanxxxx…but how can I store image URLs in database as normal string ….Plzzzz Help…..
fantastic job……
fantastic job……
Awesom……… LIKE Always……
Preetuy Coollllll Actuallyyyy….
Keep it up…….
Keep sharing your knowledge……….
Bleave me u will go veryyyyy far….
again great……………
Sir!!! thanks for nice tutorial its really usefull!!!
Sir!!! thanks for nice tutorial its really usefull!!!
Thanks for useful information.
Thanks for useful information.
Thanks 4 all your tutorials, Sub aik sey bher ker aik hain, Sir , also include tutorial for windows application, PictureBox to sql Server.
Once Again, Thank you very much.
May Allah bless you (Amen)
What a great informative tutorial
Sir, Great article please we need another article —
Display BLOB (you have saved in this article) in DataList or ListView …. Product Catalog Like scenario
Sir, Great article please we need another article —
Display BLOB (you have saved in this article) in DataList or ListView …. Product Catalog Like scenario
What a great informative tutorial