If you have been keeping yourself up-to-date with the latest development trends in the .NET world, then you must have heard about Blazor by now. There is currently a lot of hype for Blazor in the .NET community and the most common reason for this hype is that it has introduced something which most .NET developers were dreaming from decades and that is the ability to run C# not only on the server but also in the browser. Blazor allows us to build interactive web apps using HTML, CSS, and C# instead of JavaScript. In this tutorial, I will cover the basic concepts of Blazor and will give you an overview of different hosting models available for Blazor. I will also cover the pros and cons of each hosting model so that you can decide the best hosting model for your next Blazor project.
Table of Contents
What is Blazor?
Blazor is a free, open-source, single-page apps (SPA) development framework that enables developers to build interactive web apps using C# on both servers as well as client-side. Blazor does not require any plugin to be installed on the client to execute the C#/.NET code inside a browser. It executes the .NET code using WebAssembly which is a web standard supported by all major browsers. Blazor can also run .NET code and build UI on the server and transfer only the updated DOM to clients over SignalR connections.
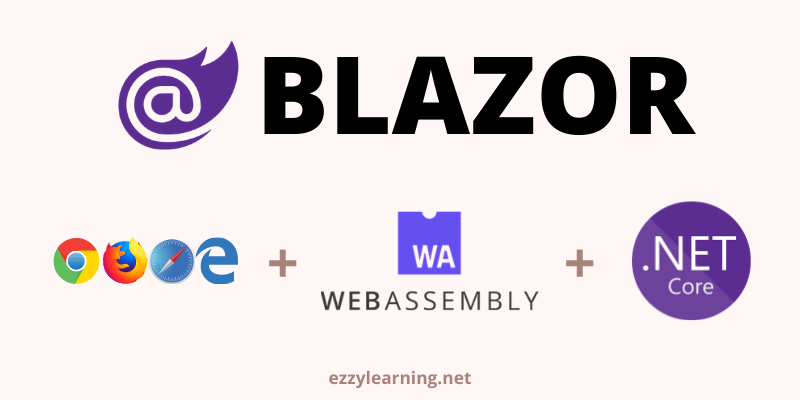
What is WebAssembly?
WebAssembly (sometimes abbreviated Wasm) is a portable binary format (low-level instructions set) designed to run on any host capable of interpreting those instructions. The main goal of WebAssembly is to allow developers to build high-performance web apps but the format is designed to be executed and integrated into other environments as well. WebAssembly is currently supported by all major browsers such as Chrome, Chrome for Android, Edge, Firefox, Safari, Opera, and many more.
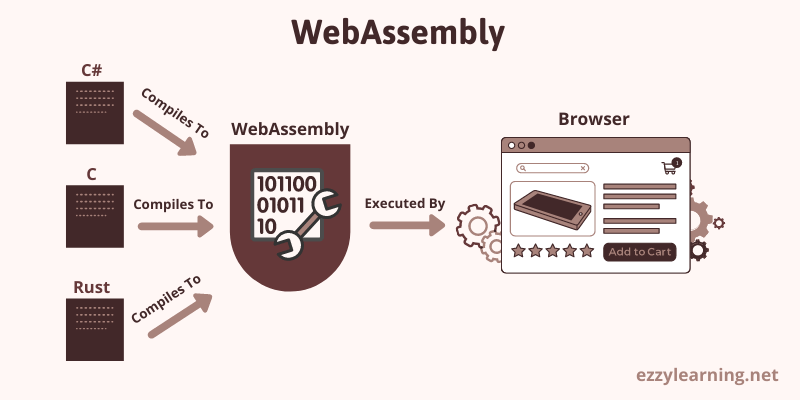
Blazor Hosting Models
The Blazor component model is the core of Blazor and it is designed in such a way that it keeps both calculating the UI changes and rendering the UI separate from each other. This is why the basic component model doesn’t change no matter what method you are using to render your apps. At the time of this writing, there are four rendering/hosting models available and they are all at different stages of development.
- Blazor Server
- Blazor WebAssembly
- Blazor Electron
- Mobile Blazor Bindings
Blazor Electron and Mobile Blazor Bindings are currently at the experimental stage and Microsoft hasn’t yet committed to shipping these hosting models so I will not discuss them in this article.
What is Blazor Server App?
Blazor Server apps run on the server where they enjoy the support of full .NET Core runtime. All the processing is done on the server and UI/DOM changes are transmitted back to the client over the SignalR connection. This two-way SignalR connection is established when the user loads the application in the browser the very first time. As your .NET code is already running on the server, you don’t need to create APIs for your front-end. You can directly access services, databases, etc., and do anything you want to do on traditional server-side technology.
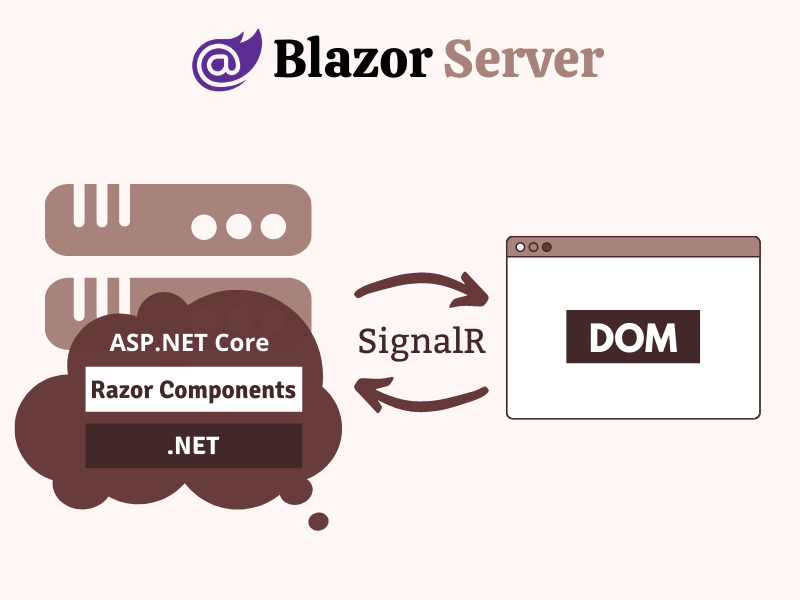
When to use Blazor Server
- When you want to run your apps on the full .NET Core runtime
- When you want to keep your apps initial download size very small
- When you want to keep your apps start-up time very fast
- When you want to keep your app’s code on the server and don’t want it to be downloaded to the client.
- When you want a fast development cycle for your apps with almost no learning curve for existing .NET developers
- When you want to make your apps search-engine friendly
- When you want your app to run on old browsers with no dependency on WebAssembly
- When you want to debug your .NET code in Visual Studio as any normal .NET app
- When you want to build intranet or low demand public-facing apps
When not to use Blazor Server
- When your apps are running in high latency environments
- When you want your app to work offline without a constant SignalR connection to the server
- When you don’t want to increase your server resources to handle a large amount of connected SignalR clients.
What is Blazor WebAssembly App?
This hosting model is a direct competitor of modern and popular SPA frameworks such as Angular, Vue, and React and this is the main reason most developers are interested to learn Blazor. It allows developers to write all front-end UI logic in C# instead of JavaScript. In this hosting model, application DLLs, any dependencies, and a small size Mono .NET runtime are downloaded to the client in the first request. Once everything is available at the client, the Mono runtime loads and executes the application code. Blazor WebAssembly programs can be written in other languages such as C, C#, etc., and then they are compiled to WebAssembly bytecode.
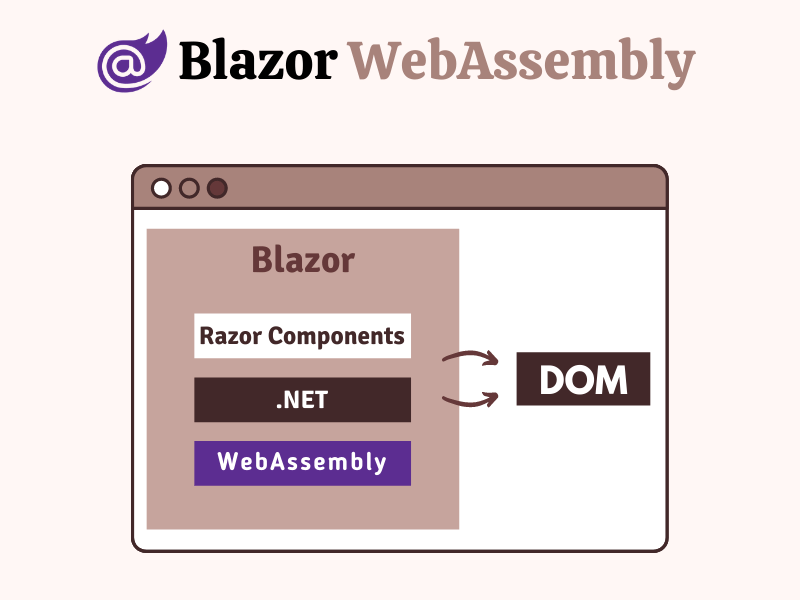
When to use Blazor WebAssembly
- When you want to compile your entire app into static files and serve them to clients with no need for a .NET runtime on the server. This means your back-end can be written in PHP, Node, or Rails and it can serve the front-end app written in Blazor.
- When you want to build apps that can run offline on the client without a constant connection to the server
- When you want to shift processing to the client and want to reduce the load on the server
- When you want to share code and libraries between client and server
When not to use Blazor WebAssembly
- When you can’t compromise on payload due to so many files/DLLs downloading to the client
- When you can’t compromise on slow start-up time especially on poor internet connections
- When you can’t compromise on the fact that the app has to operate in the browser sandbox with all security restrictions.
To understand more about Blazor hosting models let’s create Blazor Server and Blazor WebAssembly apps in Visual Studio 2019.
Creating Blazor Server App in Visual Studio 2019
Open Visual Studio 2019 Community Edition and click Create a new project option. From the list of available templates choose the Blazor App template and click Next.
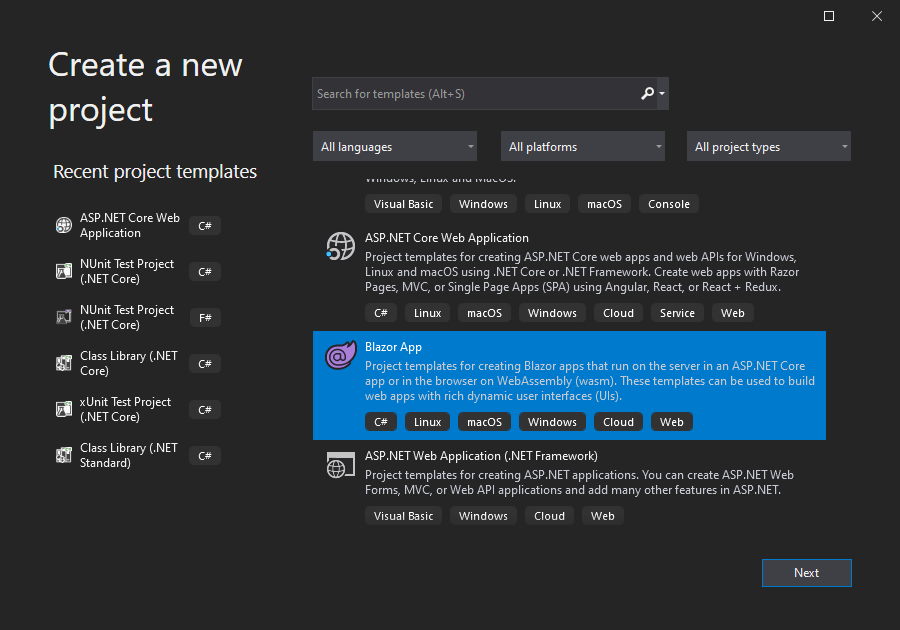
Provide the project name such as BlazorServerApp and click Next. You will the following dialog asking you to choose the type of Blazor app you want to create. We are creating the Blazor Server app so choose Blazor Server App and click Create button.
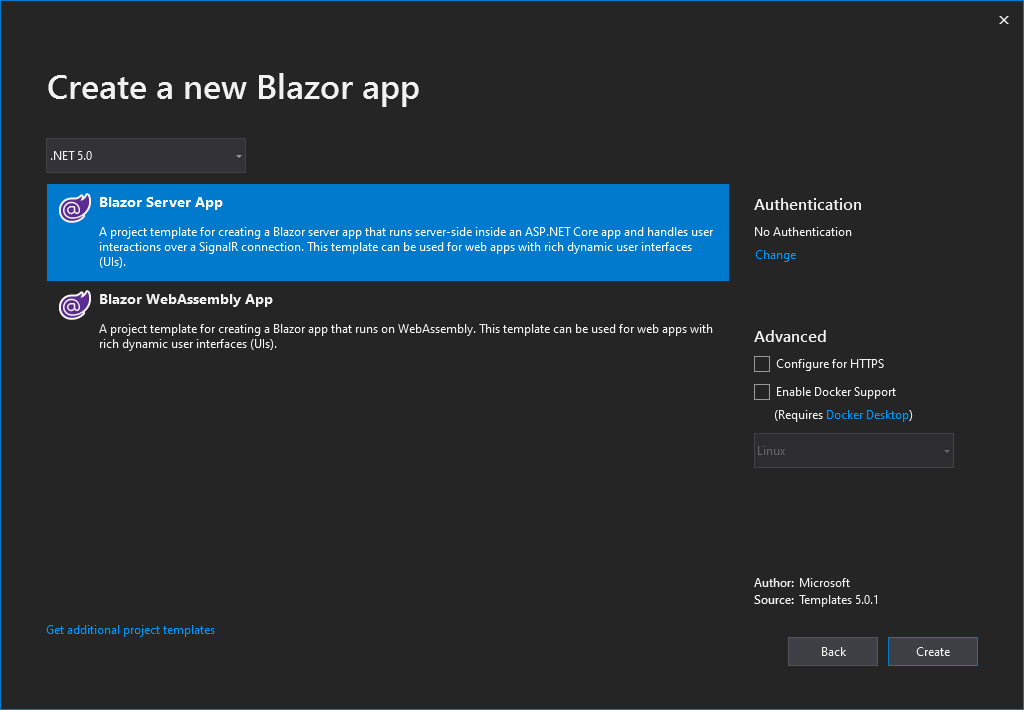
Visual Studio will create a Blazor Server App for us with the following folders and files shown in the Solution Explorer.
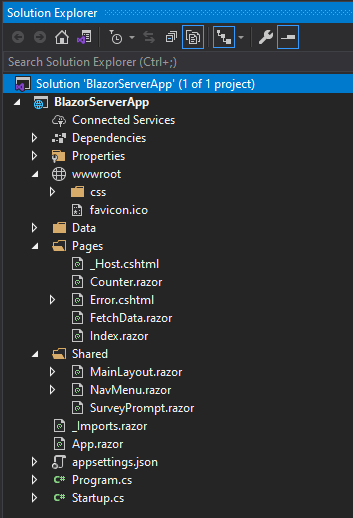
Let’s discuss some of the important files and folders available in the Blazor Server App.
Program.cs
This file contains the Main method which is the entry point of the project. The main method calls the CreateHostBuilder method which configures the Default ASP.NET Core Host for our app.
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
}
Startup.cs
This is the same file we use in standard ASP.NET Core projects. The important thing to note is that the ConfigureServices method is calling the AddServerSideBlazor method. This method adds services related to Blazor Server Apps.
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
services.AddServerSideBlazor();
services.AddSingleton<WeatherForecastService>();
}
We also have the following two important lines in Configure method. The MapBlazorHub method configures SignalR Hub endpoints required for Blazor Server App. The MapFallbackToPage method will map all those requests to _Host page which are not mapping with any controllers, razor pages, etc. This will allow all dynamic content requests to route to the SPA framework instead of throwing 404 Not Found.
app.UseEndpoints(endpoints =>
{
endpoints.MapBlazorHub();
endpoints.MapFallbackToPage("/_Host");
});
_Host.cshtml
This is the root page of the application and every Razor component/page will render within this host page. It has basic HTML elements such as html, head and body, and some special elements. Please note that Blazor is a component-based framework and everything in Blazor is a component. The <component> specifies where we want to render the root component of the application.
<component type="typeof(App)" render-mode="ServerPrerendered" />
This file is also injecting the blazor.server.js file at the end and this JavaScript file has the code to setup SignalR connection to the server. This connection is established as soon as the app loads in the browser and then it is used for real-time communication between the server and the client browser. If you want to learn more about SignalR then please read my post Display Live Sports Updates using ASP.NET Core SignalR
<script src="_framework/blazor.server.js"></script>
App.razor
This is the main component of Blazor App and its main job is to intercept the route and render either Found or NotFound components. It renders Found component if it finds a component matching with the route and renders the NotFound component if no matching component is found.
<Router AppAssembly="@typeof(Program).Assembly" PreferExactMatches="@true">
<Found Context="routeData">
<RouteView RouteData="@routeData" DefaultLayout="@typeof(MainLayout)" />
</Found>
<NotFound>
<LayoutView Layout="@typeof(MainLayout)">
<p>Sorry, there's nothing at this address.</p>
</LayoutView>
</NotFound>
</Router>
MainLayout.razor
MainLayout file contains the application’s main layout and its markup can be shared with multiple Razor components. This layout component normally contains the common UI elements of the application such as header, menu, footer, sidebar, etc. The default MainLayout generated for us has a sidebar that renders the NavMenu component and it is also using Razor syntax @Body to specify the location in the layout markup where the contents of other components will render.
@inherits LayoutComponentBase
<div class="page">
<div class="sidebar">
<NavMenu />
</div>
<div class="main">
<div class="top-row px-4">
<a href="https://docs.microsoft.com/aspnet/" target="_blank">About</a>
</div>
<div class="content px-4">
@Body
</div>
</div>
</div>
wwwroot folder
This folder contains static files such as images, fonts, icons, CSS and JavaScript files, etc.
Pages and Shared Folders
This folder contains the _Host.cshtml file we discussed earlier as well as few Razor components. Blazor App is a collection of Razor components that have the .razor extension. Some of these components are called routable components because they are accessible using their routes. For example, the following Index.razor component will render when we will navigate to the application root URL. The URL is specified using the @page directive on top of the Index.razor component.
Index.razor
@page "/"
<h1>Hello, world!</h1>
Welcome to your new app.
<SurveyPrompt Title="How is Blazor working for you?" />
Note that the above page is also using a child component SurveyPrompt which is called a child component because it doesn’t have @page directive in it and it can be embedded in other components.
Pages folder also has some other razor components in it which are all accessible using their routes specified on top of the file. For example, the Counter component will render when we will navigate to /counter path. Similarly, the FetchData component will render using /fetchdata path.
Razor Server app also has a Shared folder that contains the shared components. These components can be used by any component throughout the application just like the SurveyPrompt component we saw above. Another interesting shared component in the Shared folder is the NavMenu component that renders the top navigation bar of our Blazor Server App.
_Imports.razor
This file is similar to the _ViewImports.cshtml file we have in ASP.NET MVC Web Applications and it contains the list of namespaces we can use in different razor components. The benefit of declaring all these namespaces in _Imports.razor file is that we don’t need to import them repeatedly in each razor component.
@using System.Net.Http
@using Microsoft.AspNetCore.Authorization
@using Microsoft.AspNetCore.Components.Authorization
@using Microsoft.AspNetCore.Components.Forms
@using Microsoft.AspNetCore.Components.Routing
@using Microsoft.AspNetCore.Components.Web
@using Microsoft.AspNetCore.Components.Web.Virtualization
@using Microsoft.JSInterop
It is now time to run our Blazor Server App and see it in action in the browser. Press F5 in Visual Studio and you will see a nice looking default Blazor Server app. Try to navigate to different pages from the sidebar and also try to play with the counter on the Counter page and you will notice that there is no page refresh or post back to the server. Everything feels smooth and fast just like typical SPA and all the browser and server communication is happening using SignalR connections.
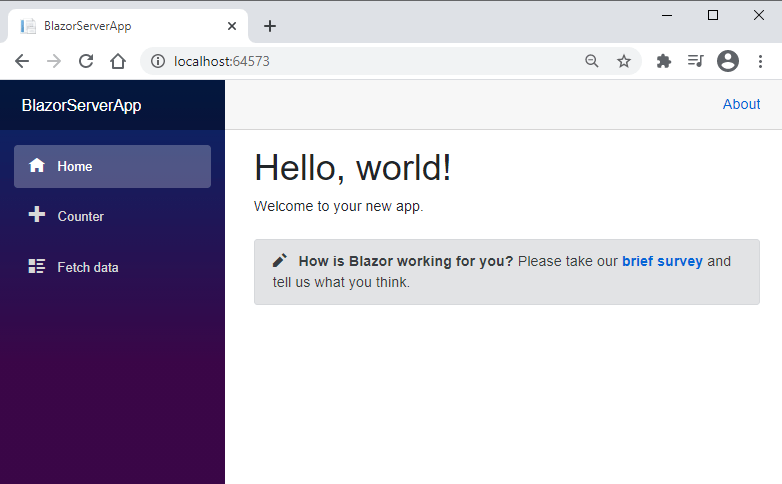
You can also open browser developer tools and you will notice that all standard CSS and JavaScript files including the blazor.server.js file are downloaded to the client and a SignalR connection is established over Web Sockets.
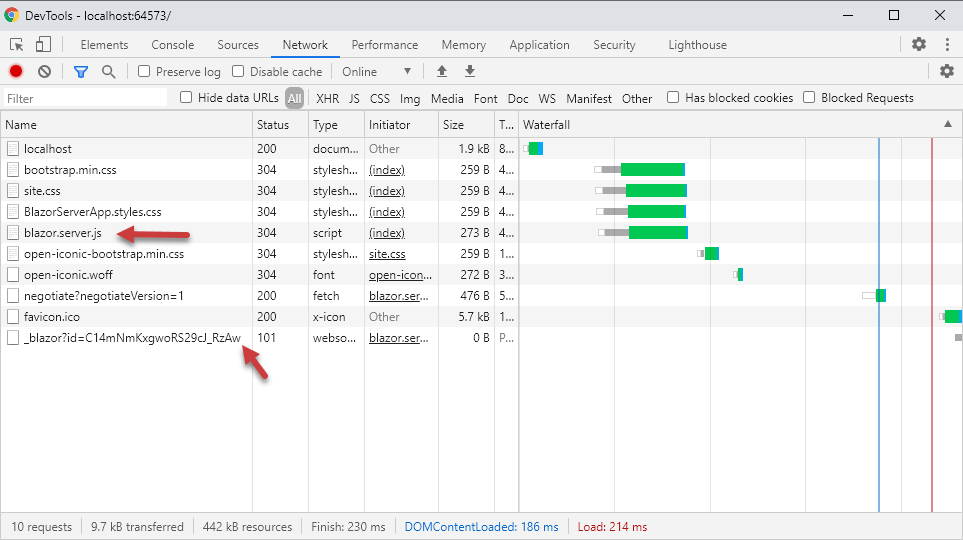
Creating Blazor WebAssembly App in Visual Studio 2019
We have learned the basics of the Blazor Server App and saw it in action in the browser. Let’s create a Blazor WebAssembly App now so that we can see the difference. Follow the same steps we mentioned above and create a new Blazor App in Visual Studio using the Blazor App template. When you will be asked to choose the type of Blazor App, you need to select Blazor WebAssembly App this time.
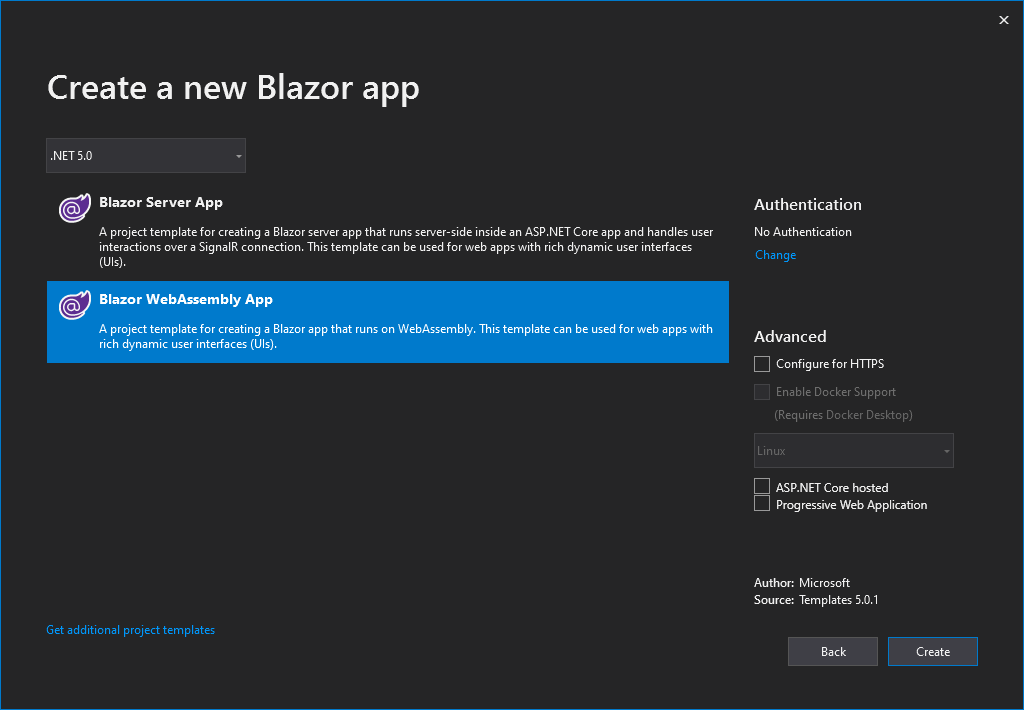
Visual Studio will create a Blazor WebAssembly App for us with the following folders and files shown in the Solution Explorer.
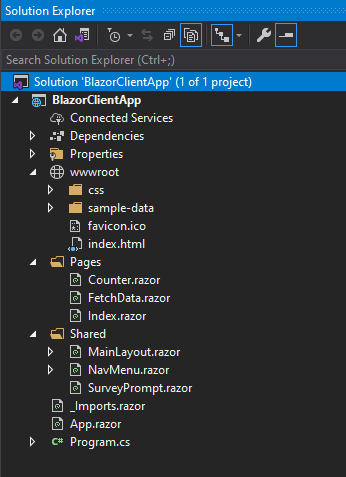
You can easily spot some of the differences between both types of apps. For example, we don’t have the following files in Blazor WebAssembly App.
- _Host.cshtml
- Error.cshtml
- Startup.cs
- appsettings.json
index.html
In Blazor WebAssembly App, we have an index.html file in wwwroot folder that serves as a root page. This file is injecting blazor.webassembly.js file at the end and this file is provided by the framework to handle download the .NET runtime, our Blazor app, and all the app dependencies. It also has code to initialize the runtime to run the app.
Program.cs
In the Blazor WebAssembly app, the root component of the app is specified in the Main method available in Program.cs file. The root component of the app is App.razor and you can see how it’s added in RootComponents collection.
public class Program
{
public static async Task Main(string[] args)
{
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("#app");
builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
await builder.Build().RunAsync();
}
}
Press F5 in Visual Studio and you will see a similar-looking Blazor WebAssembly app. Try to navigate to different pages from the sidebar and also try to play with the counter on the Counter page as you did before in Blazor Server App. Everything looks and feels the same and there are no server-side post-backs.
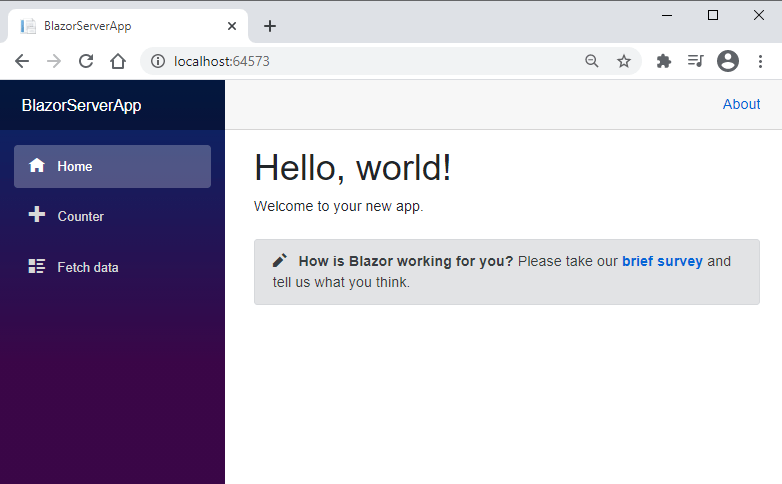
As we already know that Blazor WebAssembly apps download the app and all their dependencies on the client so you can see lots of DLLs downloaded on the client if you open browser developer tools.
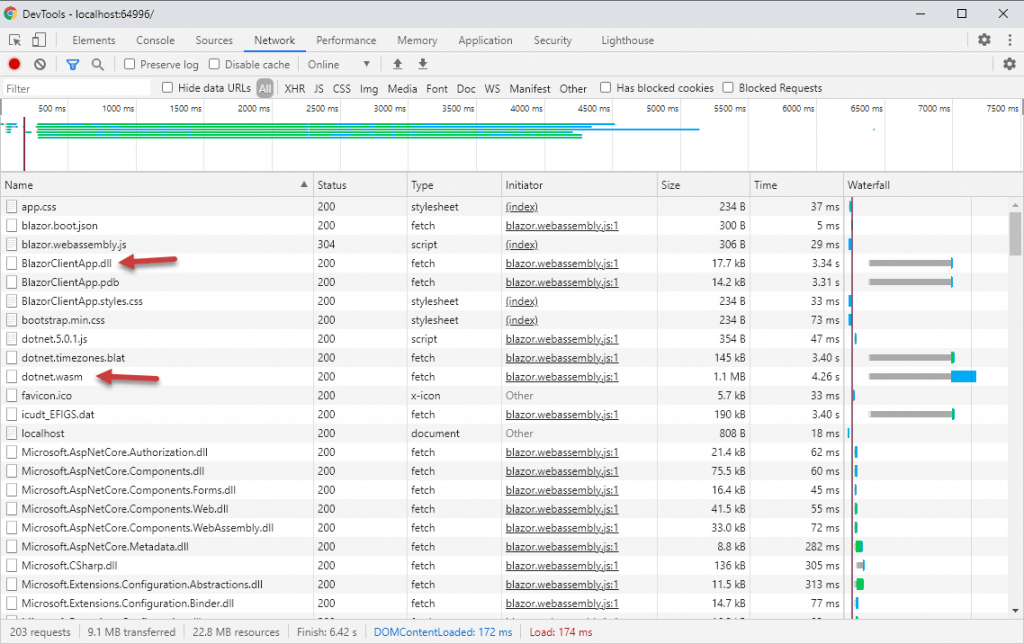
All of the above files will download only in the first request and then they will be cached in the browser. If you will refresh your page again, you will see only fewer files downloaded this time around.
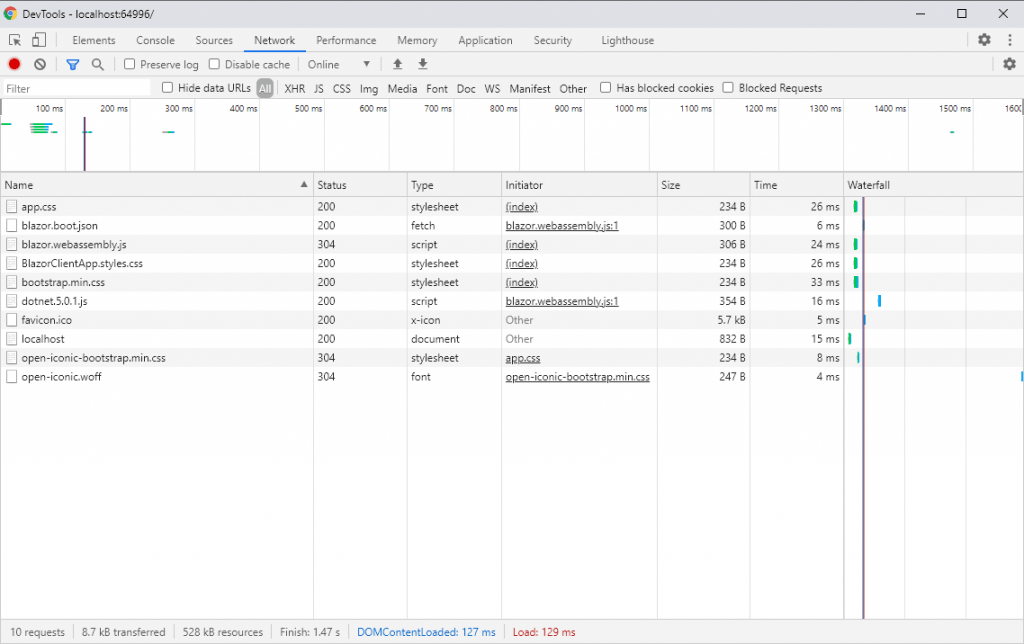
Summary
In this post, I tried to give you a basic overview of the Blazor SPA framework and we have seen two Blazor apps hosted using two different hosting models. Most of the code and files were the same in both projects because the Blazor framework relies heavily on razor components. These components are the building blocks of Blazor apps and we can build these components in a similar manner no matter what hosting model we are using. Please share this post if you liked it and spread the knowledge.
I think there is a error: You have a section heading “MainLayout.cshtml”. Shouldn’t this be “MainLayout.razor”?
Thanks bob for highlighting this mistake. It has been fixed now.
Nice article, but you forget to mention more reason when not to host client-side.. go for server-side, if you want to reuse existing business that isn’t completely async/await, uses multiple threads, unsupported language features or components like WebClient instead of HttpClient. We are in that situation… have to chose for server.