In one of my earlier tutorial I have shown you how you can call methods from custom .NET objects available in your Business Login Layer. In this tutorial, I will show you how to invoke methods which are expecting input parameters from the web form to perform their job. I will also illustrate how to pass values for these input parameters using ObjectDataSource control parameter collections.
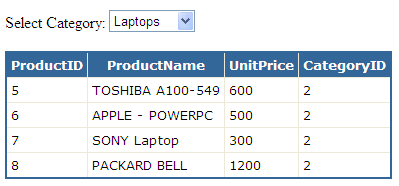
For the purpose of this tutorial, I have created two methods in custom business object. The first method returns a DataSet object filled with Categories to display in DropDownList control for user selection and the second method returns records from the Products table which are matching with the category user has selected from the DropDownList control.
public class SqlHelper
{
public DataSet GetCategories()
{
string constr = "Server=TestServer; Database=SampleDatabase; uid=test; pwd=test;";
string query = "SELECT CategoryID, CategoryName FROM Categories";
SqlConnection con = new SqlConnection(constr);
SqlCommand com = new SqlCommand(query, con);
SqlDataAdapter da = new SqlDataAdapter(com);
DataSet ds = new DataSet();
da.Fill(ds);
return ds;
}
public DataSet GetProductsByCategory(int CategoryID)
{
string constr = "Server=TestServer; Database=SampleDatabase; uid=test; pwd=test;";
string query = "SELECT ProductID, ProductName, UnitPrice, CategoryID FROM Products WHERE CategoryID = @CategoryID";
SqlConnection con = new SqlConnection(constr);
SqlCommand com = new SqlCommand(query, con);
com.Parameters.AddWithValue("@CategoryID", CategoryID.ToString());
SqlDataAdapter da = new SqlDataAdapter(com);
DataSet ds = new DataSet();
da.Fill(ds);
return ds;
}
}
Once our business object methods are ready we are ready to configure our ObjectDataSource controls on the page. The first ObjectDataSource control is configured to call GetCategories method from the business object and then it is associated with the DropDownList control with the help of DataSourceID property of DropDownList.
<asp:ObjectDataSource ID="ObjectDataSource1" runat="server"
SelectMethod="GetCategories"
TypeName="SqlHelper" />
Select Category:
<asp:DropDownList ID="DropDownList1" runat="server"
AutoPostBack="True"
DataSourceID="ObjectDataSource1"
DataTextField="CategoryName"
DataValueField="CategoryID">
</asp:DropDownList>
The second ObjectDataSource control is configured to call GetProductsByCategory method from the business object but as you noticed in the method implementation above, this method accept one input parameter of int type and to pass this input parameter value we need to use SelectParameters collection of ObjectDataSource control as shown below
<asp:ObjectDataSource ID="ObjectDataSource2" runat="server"
SelectMethod="GetProductsByCategory" TypeName="SqlHelper">
<SelectParameters>
<asp:ControlParameter ControlID="DropDownList1" Name="CategoryID"
PropertyName="SelectedValue" Type="Int32" DefaultValue="1" />
</SelectParameters>
</asp:ObjectDataSource>
In SelectParameters collection I am using ControlParameter which is setup to get the value of the input parameter from the DropDownList automatically by using its SelectedValue property. If you want to pass input parameter value from any other source such as query string, cookies or session etc, you can use other type of parameter in SelectParameters collection. The final bit here is to associate GridView control with the second ObjectDataSource control to display products on the page.
<asp:GridView ID="GridView1" runat="server" DataSourceID="ObjectDataSource2"
BorderColor="#336699" BorderStyle="Solid" BorderWidth="2px" CellPadding="4"
Font-Names="Verdana" Font-Size="10pt">
<HeaderStyle BackColor="#336699" ForeColor="White" />
</asp:GridView>
I hope you have basic idea how to call methods by passing input parameters to them by using ObjectDataSource control. The beauty of this control is that you don’t have to write single line of code for calling parametrize methods from the page. You just need to declare the parameter type in SelectParameters collection. Keep in mind that ObjectDataSource control also has InsertParameters, UpdateParameters, DeleteParameters and FilterParameters collections to call parameters methods for those operations too.
hello I am trying to do same thing. But I am getting an error as : No value given for one or more required parameters
I am using Dropdownlist and Formview control and using Businesslogic and DAL in asp.net 2.0…