AJAX is definitely the hot buzzword in the Web Application development world at the moment. AJAX which is an acronym of Asynchronous JavaScript and XML is a group of interrelated web development techniques used on the client side to create dynamic, interactive and rich internet applications. Using AJAX a web application can send and receive data from the server asynchronously in the background without interfering with the display and behavior of the page. In this tutorial I will show you how you can use ASP.NET AJAX UpdatePanel control and its features to implement AJAX behavior in your website.
Microsoft ASP.NET AJAX provides developers both server side and client side AJAX frameworks. Server side framework provides an easy way to implement AJAX functionality without having to learn JavaScript. On the other hand, client side framework provides developers tools and libraries to build pure client-side AJAX applications. If you are using ASP.NET 2.0 version you have to download and install ASP.NET AJAX from the Microsoft ASP.NET official website (www.asp.net), however if you are using ASP.NET 3.5 with Visual Studio 2008 you will find a separate category named “AJAX Extensions” on the toolbox as following figure shows:
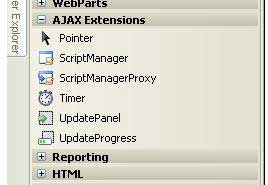
AJAX Extensions category in toolbox has five server side AJAX controls which are very easy to use as they required almost no knowledge of JavaScript or any other client side technology such as DOM or DHTML.
Table of Contents
ScriptManager
ScriptManager control is required to use any ASP.NET AJAX feature or control on the page. It manages client script libraries and JavaScript proxy classes to call web services and provides basic AJAX features such as partial page rendering and asynchronous post backs.
UpdatePanel
UpdatePanel control is the most important and widely used ASP.NET AJAX server control. It enables you to refresh selected parts of the page partially instead of refreshing the whole page with a post back. This control works with ScriptManager control and it provides you facility to perform partial page updates automatically with the help of client script.
To start this tutorial, create a new ASP.NET web form in your web application and add ScriptManager and UpdatePanel controls. For the purpose of this tutorial you need to add a RadioButtonList, Label and Image control inside UpdatePanel ContentTemplate as shown below:
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<br />
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<asp:RadioButtonList ID="RadioButtonList1" runat="server" AutoPostBack="True"
onselectedindexchanged="RadioButtonList1_SelectedIndexChanged"
BackColor="#FFFFCC" BorderColor="Black" BorderStyle="Solid" BorderWidth="2px">
<asp:ListItem Value="images/ajax_in_action.jpg">
AJAX in Action
</asp:ListItem>
<asp:ListItem Value="images/ajax_bible.jpg">
AJAX Bible
</asp:ListItem>
<asp:ListItem Value="images/understanding_ajax.jpg">
Understanding AJAX
</asp:ListItem>
</asp:RadioButtonList>
<br />
<asp:Label ID="Label1" runat="server" Font-Size="14pt">AJAX in Action</asp:Label>
<br />
<br />
<asp:Image ID="Image1" runat="server" ImageUrl="~/images/ajax_in_action.jpg" />
</ContentTemplate>
</asp:UpdatePanel>
As you can see I am adding the names and image URLs in the RadioButtonList control to allow user to select one of the book from the list. When user will select any book from the RadioButtonList, its Title and Image will be displayed on Label and Image controls which are also inside UpdatePanel ContentTemplate. You can also drag and drop these controls directly from the Visual Studio 2008 toolbox into the UpdatePanel. The following figure shows how your page will look like at design time in Visual Studio 2008.
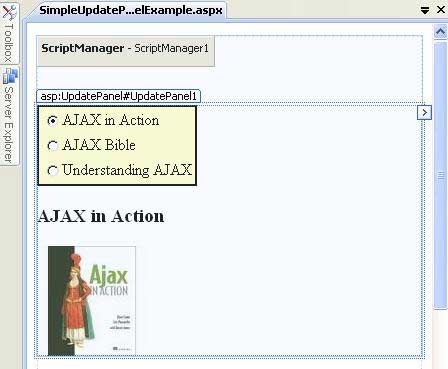
In this example I set AutoPostBack property of the RadioButtonList control to true and that’s because every time user will select a Book Title it will post back automatically to the server to execute the code written in SelectedIndexChanged event of the control as following code shows:
protected void RadioButtonList1_SelectedIndexChanged(object sender, EventArgs e)
{
Image1.ImageUrl = RadioButtonList1.SelectedValue;
Label1.Text = RadioButtonList1.SelectedItem.Text;
}
The code above is straight forward and is setting the ImageUrl and Text properties of Image and Label control from the RadioButtonList selected item. If you will run this page now and will select different book titles the book title and image will change very quickly without full ASP.NET postback. Following figure shows the output with all three book titles selected.
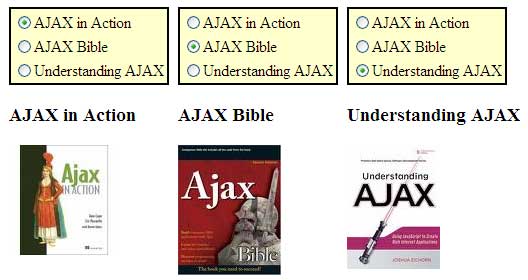
The UpdatePanel control is doing all the AJAX work in this example. It hijacks the normal postback that would happen when you select a new item in the RadioButtonList control and then perform an asynchronous AJAX postback in the background to execute server side code.
Using Triggers with UpdatePanel
By default, UpdatePanel automatically sends asynchronous postback for every child control added inside the UpdatePanel and this is because of its ChildrenAsTriggers property which is set to true. If you have placed your control outside the UpdatePanel and you sill want UpdatePanel to perform partial page updates based on that control you can use its Triggers Collection. You need to specify an asynchronous post back trigger with the ControlID and the EventName properties as shown below:
<asp:RadioButtonList ID="RadioButtonList1" runat="server" AutoPostBack="True"
OnSelectedIndexChanged="RadioButtonList1_SelectedIndexChanged"
BackColor="#FFFFCC" BorderColor="Black" BorderStyle="Solid" BorderWidth="2px">
<asp:ListItem Value="images/ajax_in_action.jpg">
AJAX in Action
</asp:ListItem>
<asp:ListItem Value="images/ajax_bible.jpg">
AJAX Bible
</asp:ListItem>
<asp:ListItem Value="images/understanding_ajax.jpg">
Understanding AJAX
</asp:ListItem>
</asp:RadioButtonList>
<asp:UpdatePanel ID="UpdatePanel1" runat="server" >
<ContentTemplate>
<br />
<asp:Label ID="Label1" runat="server" Font-Size="14pt">AJAX in Action</asp:Label>
<br />
<br />
<asp:Image ID="Image1" runat="server" ImageUrl="~/images/ajax_in_action.jpg" />
</ContentTemplate>
<Triggers>
<asp:AsyncPostBackTrigger ControlID="RadioButtonList1" EventName="SelectedIndexChanged" />
</Triggers>
</asp:UpdatePanel>
Now even if you RadioButtonList control is outside the UpdatePanel control, you will still be able to get the benefit of partial page update using UpdatePanel. You can also add multiple triggers if you want more than one control to initiate asynchronous postback.
Updating UpdatePanel Programmatically
UpdatePanel control also has UpdateMode property which can be set to Always or Conditional. By default, it is set as Always which means if any control either inside or outside the UpdatePanel control will perform asynchronous postback the UpdatePanel contents will always refresh automatically. If you will set this property to Conditional you have to update the UpdatePanel programmatically from the code using its Update() method as following example shows:
<asp:UpdatePanel ID="UpdatePanel1" runat="server" UpdateMode="Conditional">
<ContentTemplate>
<asp:RadioButtonList ID="RadioButtonList1" runat="server" AutoPostBack="True"
onselectedindexchanged="RadioButtonList1_SelectedIndexChanged"
BackColor="#FFFFCC" BorderColor="Black" BorderStyle="Solid" BorderWidth="2px">
<asp:ListItem Value="images/ajax_in_action.jpg">
AJAX in Action
</asp:ListItem>
<asp:ListItem Value="images/ajax_bible.jpg">
AJAX Bible
</asp:ListItem>
<asp:ListItem Value="images/understanding_ajax.jpg">
Understanding AJAX
</asp:ListItem>
</asp:RadioButtonList>
<br />
<asp:Label ID="Label1" runat="server" Font-Size="14pt">AJAX in
Action</asp:Label>
<br />
<br />
<asp:Image ID="Image1" runat="server" ImageUrl="~/images/ajax_in_action.jpg" />
</ContentTemplate>
</asp:UpdatePanel>
The code behind file of the above page is shown below in which I am using Update method of UpdatePanel programmatically.
protected void RadioButtonList1_SelectedIndexChanged(object sender, EventArgs e)
{
Image1.ImageUrl = RadioButtonList1.SelectedValue;
Label1.Text = RadioButtonList1.SelectedItem.Text;
UpdatePanel1.Update();
}
I hope this tutorial on UpdatePanel control will give you basic idea of ASP.NET AJAX server side controls. There are so many other things to learn when you are creating AJAX based ASP.NET website so keep visiting my website regularly for some more tutorials in future.
tanksssssss
Asalam-o-Alaikum
Sir is there any difference between “Partial Page Rendering” and “Asynchronous Post backs” or they are more or less same things?
In “Using Triggers with UpdatePanel” Section also works if we set ChildrenAsTriggers property to true
and update mode to conditional why?
ChildrenAsTriggers property specify whether children can trigger the
postback. By default this property is true and cause all the controls
inside UpdatePanel postback automatically.
Triggers collection is used to trigger async postbacks from the controls
hosted outside update panel.
UpdateMode=Conditional allows you to update the UpdatePanel
programmatically using Update() method available in UpdatePanel.