Paging large database result sets is very important and common feature in modern websites. For ASP.NET developers, Microsoft has build paging functionality into the GridView control by default and developers just have to set some simple properties true and that’s all they need to have paging in their websites. Many developers prefer Repeater or DataList control over GridView due to its large overhead. In this tutorial, I will show you how you can do paging using light weight ASP.NET Repeater control and PagedDataSource class.
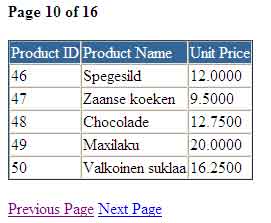
The PagedDataSource class
This class is available in System.Web.UI.WebControls namespace, so you don’t have to import any additional namespace to the ASP.NET page. This class provides you facility to add paging functionality to DataList, Repeater or any other control. It encapsulates all the functionality of GridView paging and if you have ever used GridView paging, you will find the following properties of this class very familiar to you.
- AllowPaging – This Boolean property determines whether the paging should be enabled or disabled. By default it is set to false.
- PageSize – This property defines how many records to display on a page. The default value is 10.
- CurrentPageIndex – This property returns or set the current page number. By default it returns 0.
- PageCount – This property returns the total number of pages
- DataSource – The value of this property will be the source of the data you want to page. i.e. DataTable.
- IsFirstPage – This property returns true or false depending on whether the current page is the first page.
- IsLastPage – This property returns true or false depending on whether the current page is the last page.
Now you have basic idea of important properties of PagedDataSource class I am showing you live working example how to use this class to implement paging with Repeater control. Following code is HTML source of the ASP.NET page showing you a simple Repeater control with Header, Footer and ItemTemplate. All three templates are creating a simple HTML table to make it look like GridView control.
<asp:Label ID="Label1" runat="server" Font-Bold="True" />
<br />
<br />
<asp:Repeater ID="Repeater1" runat="server">
<HeaderTemplate>
<table border="1" style="border-color: #336699;" cellspacing="0">
<tr style="background-color:#336699; color:White;">
<td>Product ID</td>
<td>Product Name</td>
<td>Unit Price</td>
</tr>
</HeaderTemplate>
<ItemTemplate>
<tr>
<td><%# Eval("ProductID") %></td>
<td><%# Eval("ProductName") %></td>
<td><%# Eval("UnitPrice") %></td>
</tr>
</ItemTemplate>
<FooterTemplate>
</table>
</FooterTemplate>
</asp:Repeater>
<br />
<asp:HyperLink ID="linkPrev" runat="server">Previous Page</asp:HyperLink>
<asp:HyperLink ID="linkNext" runat="server">Next Page</asp:HyperLink>
You must notice Label control and two HyperLink controls. These controls are not part of the Repeater control and are placed outside Repeater control. The Label control will show user current page and total number of pages and hyper link controls will provide Next and Previous page functionality.
Following is the C# code behind file for the above page. For brevity I am putting all the code in Page_Load event of the page.
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
string constr = "Server=.\\SQLEXPRESS; Database=NORTHWND; uid=test; pwd=test";
string query = "SELECT * FROM Products";
SqlDataAdapter da = new SqlDataAdapter(query, constr);
DataTable table = new DataTable();
da.Fill(table);
PagedDataSource pds = new PagedDataSource();
pds.DataSource = table.DefaultView;
pds.AllowPaging = true;
pds.PageSize = 5;
int currentPage;
if (Request.QueryString["page"] != null)
{
currentPage = Int32.Parse(Request.QueryString["page"]);
}
else
{
currentPage = 1;
}
pds.CurrentPageIndex = currentPage - 1;
Label1.Text = "Page " + currentPage + " of " + pds.PageCount;
if (!pds.IsFirstPage)
{
linkPrev.NavigateUrl = Request.CurrentExecutionFilePath + "?page=" + (currentPage - 1);
}
if (!pds.IsLastPage)
{
linkNext.NavigateUrl = Request.CurrentExecutionFilePath + "?page=" + (currentPage + 1);
}
Repeater1.DataSource = pds;
Repeater1.DataBind();
}
}
Explanation
I am using Microsoft’s very popular Northwind database for this example and first few lines in the above code are filling a DataTable object by using ADO.NET SqlDataAdapter object
string constr = "Server=.\\SQLEXPRESS; Database=NORTHWND; uid=test; pwd=test";
string query = "SELECT * FROM Products";
SqlDataAdapter da = new SqlDataAdapter(query, constr);
DataTable table = new DataTable();
da.Fill(table);
The following code creates an instance of PagedDataSource class and sets some basic properties.
PagedDataSource pds = new PagedDataSource();
pds.DataSource = table.DefaultView;
pds.AllowPaging = true;
pds.PageSize = 5;
The following code is checks the current page number in query string and then sets the CurrentPageIndex property of PagedDataSource accordingly.
if (Request.QueryString["page"] != null)
{
currentPage = Int32.Parse(Request.QueryString["page"]);
}
else
{
currentPage = 1;
}
pds.CurrentPageIndex = currentPage - 1;
Label1.Text = "Page " + currentPage + " of " + pds.PageCount;
Following code updates Next and Previous pages links dynamically. It also checks whether the current page is first or last page.
if (!pds.IsFirstPage)
{
linkPrev.NavigateUrl = Request.CurrentExecutionFilePath + "?page=" + (currentPage - 1);
}
if (!pds.IsLastPage)
{
linkNext.NavigateUrl = Request.CurrentExecutionFilePath + "?page=" + (currentPage + 1);
}
Last two lines in the code are binding PagedDataSource instance with Repeater control.
Repeater1.DataSource = pds; Repeater1.DataBind();
I hope some ASP.NET developers will find this tutorial very useful and I am sure for many of you PagedDataSource is totally new object. .NET Framework is full of many useful classes to make your life easier. It’s just a matter of knowing and working with them. I will recommend you to visit .NET Framework SDK documentation regularly to keep yourself up to date.
And for MVC ?
VERY USEFUL
Thanks for posting. Its really helpful for me. I saved this as bookmark. Thanks Again.
thank you for this codes. my thesis is complete
so good
thank you so much
Very Easy And Nice Tutorial Sir Thanks a Lot
dear sir,
very useful example.
Regards,
P.Prathip
Very well layed out. I like the fact the code is very light without a bunch of “extras” in it. If one has a general understanding of the concept, this code example leaves lots of “obvious” room for your own customization. VERY NICE indeed.
Excellent.
Code explanation technique is awesome. Thanks a lot.
Very detailed explanation of PagedDataSource, thank you so much
Very easy to understand. Even beginner also can able to easily understand the code.
Very thanks
i realy like this site and tutorial too logic used is simple, easy to understand. an has proved useful to me.
thanks a lot
dear Waqas Anwar
thank you so much
that was so great and helpful for me!
This is very usefull.
Hi!
this good way to use this
Dear Sir,
It is a very good tutorial.And very easy to understand because you explain every thing.Thank You Sir
Hello
My pagedatasource always return 1st two records each time I call the function with current page index (where i ve placed the pagedatasource code)
pagesize is 2 but records count from datasource is 6
Respected Sir,
I have no words to say thanks against this detailed tutorial. this is most helpful tutorial specially for me.