Displaying records in a tabular format is very common functionality of modern websites. Almost all the websites you visit these days have data to display in a table or datagrid and most of them also required the functionality of sorting records based on any column. Last week I had a chance to work with one of a popular JQuery plugin called Tablesorter and I was so impressed that I decided to write a full tutorial on it. In this tutorial, I will show you how you can use JQuery Tablesorter plugin with ASP.NET GridView control to provide client side sorting functionality to your site visitors.
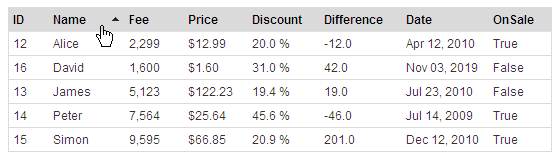
f you want to play with the Tablesorter JQuery plugin yourself with all the customized options, make sure you download the latest version of Tablesorter plugin from the following URL.
Create a new ASP.NET website and drag a GridView control on the page. Disable the automatic columns generation by using AutoGenerateColumns property and add some bound fields inside the GridView Columns collection. For this tutorial, make sure your GridView control HTML markup looks similar to the following:
<asp:GridView ID="GridView1" runat="server" CellPadding="4" ForeColor="#333333"
GridLines="Horizontal" Font-Size="9pt" Font-Names="Arial"
AutoGenerateColumns="False" BorderColor="#dadada"
BorderStyle="Solid" BorderWidth="1px">
<Columns>
<asp:BoundField DataField="ID" HeaderText="ID"
ItemStyle-Width="40" />
<asp:BoundField DataField="Name" HeaderText="Name"
ItemStyle-Width="80" />
<asp:BoundField DataField="Fee" DataFormatString="{0:n0}" HeaderText="Fee"
ItemStyle-Width="60" />
<asp:BoundField DataField="Price" DataFormatString="{0:c}"
HeaderText="Price" ItemStyle-Width="60" />
<asp:BoundField DataField="Discount" DataFormatString="{0:p1}"
HeaderText="Discount" ItemStyle-Width="70" />
<asp:BoundField DataField="Difference" DataFormatString="{0:n1}"
HeaderText="Difference" ItemStyle-Width="80" />
<asp:BoundField DataField="Date" DataFormatString="{0:MMM dd, yyyy}"
HeaderText="Date" ItemStyle-Width="100" />
<asp:BoundField DataField="OnSale" HeaderText="OnSale"
ItemStyle-Width="60" />
</Columns>
</asp:GridView>
As you can see bound fields are bound with columns such as ID, Name, Fee and so on. These columns are coming from a temporary DataTable object which I have filled and bind with GridView in the Page Load event. Following code shows the Page Load event and the code required to fill DataTable.
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
BindData();
}
}
private void BindData()
{
int[] ids = {12, 13, 14, 15, 16};
string[] names = {"Alice", "James", "Peter", "Simon", "David"};
int[] fee = { 2299, 5123, 7564, 9595, 1600 };
decimal[] prices = { 12.99m, 122.23m, 25.64m, 66.85m, 1.60m };
decimal[] discounts = { 0.2m, 0.194m, 0.4564m, 0.209m, 0.310m };
decimal[] differences = { -12m, 19.4m, -45.64m, 200.9m, 41.60m };
string[] dates = { "04-12-2010", "07-23-2010", "07-14-2009", "12-12-2010", "11-03-2019" };
bool[] onSale = { true, false, true, true, false };
DataTable table = new DataTable();
table.Columns.Add("ID", typeof(System.Int32));
table.Columns.Add("Name", typeof(System.String));
table.Columns.Add("Fee", typeof(System.Decimal));
table.Columns.Add("Price", typeof(System.Decimal));
table.Columns.Add("Discount", typeof(System.Decimal));
table.Columns.Add("Difference", typeof(System.Int32));
table.Columns.Add("Date", typeof(System.DateTime));
table.Columns.Add("OnSale", typeof(System.Boolean));
for (int i = 0; i < 5; i++)
{
DataRow row = table.NewRow();
row["ID"] = ids[i];
row["Name"] = names[i];
row["Fee"] = fee[i];
row["Price"] = prices[i];
row["Discount"] = discounts[i];
row["Difference"] = differences[i];
row["Date"] = DateTime.Parse(dates[i]);
row["OnSale"] = onSale[i];
table.Rows.Add(row);
}
GridView1.DataSource = table;
GridView1.DataBind();
GridView1.UseAccessibleHeader = true;
GridView1.HeaderRow.TableSection = TableRowSection.TableHeader;
}
Before you build and test your web page, add the following CSS styles inside page head section to add some spice to your GridView.
<style type="text/css">
th
{
cursor:pointer;
background-color:#dadada;
color:Black;
font-weight:bold;
text-align:left;
}
th.headerSortUp
{
background-image: url(images/asc.gif);
background-position: right center;
background-repeat:no-repeat;
}
th.headerSortDown
{
background-image: url(images/desc.gif);
background-position: right center;
background-repeat:no-repeat;
}
td
{
border-bottom: solid 1px #dadada;
}
</style>
Add the following JQuery and Tablesorter javascript files references inside page head section.
<script src="scripts/jquery-1.4.3.min.js" type="text/javascript"></script>
<script src="scripts/jquery.tablesorter.min.js" type="text/javascript"></script>
Finally call the tablesorter function on GridView to make your GridVeiw sortable.
<script type="text/javascript">
$(document).ready(function() {
$("#GridView1").tablesorter();
});
</script>
You can see how easy is to add client side sorting functionality to your GridView using the Tablesorter plugin. You just need to call a single function Tablesorter to implement basic sorting. However, there are many other options to customize the sorting functionality, and I will recommend you to visit the Tablesorter plugin home page to read the documentation for all the available options.
how fixed footer
Hi,
Your code is very simple and clear. But when i am using pagination.. My Page number is also getting sort. Can you please provide us any solution for this.
Thanks & Regards
Zubair Khan
Please note that the following Javascript code
$(“#GridView1”).tablesorter();
does not work if you are using Masterpages.
You need to modify it as
$(“#<%=GridView1.ClientID%>”).tablesorter();
This is incredible. Great work. This was a huge help. I never would have figured it out without you!
When page is postback after any button then the sorting is not working…. can you please provide a solution.
Your code is works fine. i when paging is included then it is not working.
Please let me know how this code is work with paging.
Sorting not working on user control
I am impressed with the effort you have so obviously put into this content. I am also impressed with your point of view on this topic, especially since you have made your points so clear.
Sorting is not working after page postback.
Sir may Allah bless you. You are among the most sincere teacher i have in my life..thanks a ton for this..
Your Student
Furqan Zafar
hello friends, actually i am trying to sort my asp:datalist by using jquery. so can anybody please suggest me something that how can i do the same.
Thanks.
nice tutorial…but if u include pagination the the pagination values get shifted from top to bottom…ne solution???
alpha pagination along with pagination and sorting would be gr8???
Good work bro, thumbs up!