Android ListView control is designed to display a list of items to the user and the most common action users perform with the ListView is the item selection by just tapping any particular item in the ListView. ListView allows developers to handle user tapping by attaching the OnItemClickListener and overriding the onItemClick event. In most cases, when user click any item in the ListView, a new android activity opens that shows the details related to the selected item. To implement such scenario the knowledge of Android Intents and activity to activity communication is required. I will show you that in my future tutorials. In this tutorial, I will demonstrate you how you can handle the click events in ListView.
Create a new Android project in Eclipse with MainActivity as your default Activity and main.xml as your default layout file. Declare a ListView control in your main.xml layout file as shown in the following code.
Table of Contents
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ListView
android:id="@+id/listView1"
android:layout_height="fill_parent"
android:layout_width="fill_parent" />
</LinearLayout>
The above code is using simple LinearLayout with vertical orientation, and a ListView is declared to cover the entire width and height of the parent container using the fill_parent as the value of both android:layout_height and android:layout:width properties. ListView also has a unique id listView1 that will be used in the MainActivity to reference the ListView control.
Before I show you the MainActivity code, you need to create a java class whose objects you will bind with the ListView. Add a new class Product in your Android project and add the following code in it.
Product.java
public class Product {
private int id;
private String name;
private double price;
public Product(){
super();
}
public Product(int id, String name, double price) {
super();
this.id = id;
this.name = name;
this.price = price;
}
@Override
public String toString() {
return this.id + ". " + this.name + " [$" + this.price + "]";
}
}
Inside the MainActivity,an array of Product objects is created and an ArrayAdapter is used to bind the array with the ListView. To handle the ListView item click events you need to use setOnItemClickListener method of ListView class. This method requires an object of the class that is implementing OnItemClickListener interface. In this particular case, I have decided to create implementation on the fly using java anonymous class. To implement OnItemClickListener interface, the method onItemClick must need to be defined as shown in the code below. This method will be fired everytime user will click any item in the ListView. By default, ListView uses the TextView objects to render every item in the List so the onItemClick implementation shown here simply cast the internal view to TextView and gets the string displayed on the TextView using the getText() method of the TextView class. Finally, the selected item text is displayed using the Android Toast class.
MainActivity.java
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
ListView listView1 = (ListView) findViewById(R.id.listView1);
Product[] items = {
new Product(1, "Milk", 21.50),
new Product(2, "Butter", 15.99),
new Product(3, "Yogurt", 14.90),
new Product(4, "Toothpaste", 7.99),
new Product(5, "Ice Cream", 10.00),
};
ArrayAdapter<Product> adapter = new ArrayAdapter<Product>(this,
android.R.layout.simple_list_item_1, items);
listView1.setAdapter(adapter);
listView1.setOnItemClickListener(new OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position,
long id) {
String item = ((TextView)view).getText().toString();
Toast.makeText(getBaseContext(), item, Toast.LENGTH_LONG).show();
}
});
}
}
Run your project and click any item in the ListView. You will be able to see the selected item text in a Toast message as shown in the screenshot below.
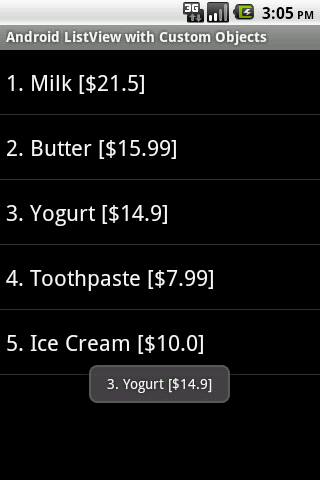
sir when i click item i recieved errors
Thanks so much man <3
thank you so much for this tutorial.
well, how to write the total amount when i click item
nice
How to make each listview clicked to jump another activity ?
Thank you very much ! This was the final answer to a day and a half of searching why my onClick event didn’t get executed.
Thankyou it’s working. But i have my data in Listview which is coming from the SQL database how will i give onItemClick event to it. plzz help me out.
Thanks Man…Its working fine but you forget to write impliments onItemClickListener in your code.
I have to select first item on long click and further items with just single click…How can i do that?
Thanks for the tutorial, my scenario is a bit different; I have a text file and i want to provide a menu on the list view so when user clicks eg. Apple on the list view it reads the text file contents and display it in a text in a new activity. How can i achieve that? Any tips. I am a newby here.
Thank you, helped me along.
Mick
can we intent a blank page through onitemclicklister for every item of list in place of toast. if its possible pls tell me how
Thanks dude. It helped a lot….
how to take the price only and display it in toast.?
Very simple and it Works!! Thank you!!
Really USEFUL….Many thanks
Needless to say, your code helped me with mine. Thanks Waqas!
NICE ONE DEAR
Sir !! I need your next tutorial….. where is your next tutorial…. thanx Sir….
Beautiful . I have to praise because you paid me..
V nice and easy to understand ..
its a very nice post…..
very nice post…..
thank you!!!
Can you tell me how can i listView1.setOnItemClickListener(new OnItemClickListener() add it? i am confused here you said interface. How can i add interface or class?
can you guide me more?
sir can we do toast along with an intent in this code
Thanks!
thanx
is there any sample code for Listview having Checkbox, and saving checkbox status is save/retrieved from database
thank you so much! all the freaking google was been unable to help me, and you helped!
Ok, but how to extract only ID in toast?
excellent!
Easy to understand. Thanks.
Thanks for the listview onitemclick tutorial–very good