Flickr is world’s largest and most popular photos hosting and sharing website, which provides a platform to many amateur and professional photographers to share their work with people all over the world. It has millions of photos in its database, and it provides photo feeds to thousands of websites and blogs every day. If you are an experience developer you can use Flickr API to spice up any website or blog with high quality photos, and if you scared off Flickr API then don’t worry Flickr has public photos feed available to you, which returns photos based on matching keywords in a pretty straight forward manner. In this tutorial, I will show you how you can mix the magic of JQuery and JSON with Flickr photo feeds to retrieve and display photos ASP.NET.
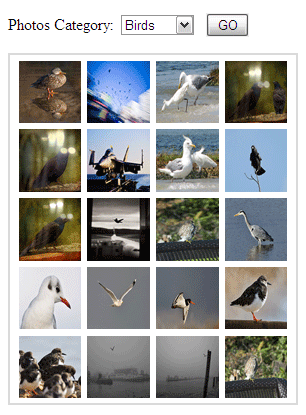
JQuery is the world’s most famous JavaScript library for web developers, and you can guess its popularity from its list of customers as Facebook, Twitter, Google, Sony, Dell, Microsoft and many more are using JQuery on their websites. It not only provides easy to use API to manipulate page contents but also provide features to communicate with the server using latest technologies such as AJAX, XML and JSON.
If you are not familiar with JQuery and JSON and don’t know what they are how to use them in ASP.NET I will recommend you to read my following tutorials before you start reading this tutorial.
Introducing JQuery for ASP.NET Developers
Using JQuery, AJAX, JSON and ASP.NET Web Services
If you are interested in using Flickr Photos APIs then visit the following link to read about API, sample codes and documentation.
http://www.flickr.com/services/api
Flickr photo feed URL documentation is available at following URL:
http://www.flickr.com/services/feeds/docs/photos_public
Following is the URL Flickr provides to developers to query public photos from the Flickr database:
http://api.flickr.com/services/feeds/photos_public.gne
In this tutorial, I will use the above Flickr feeds URL with parameters like tags, tagmode and format using the JQuery getJSON() method which will send AJAX request to the Flickr server and will retrieve photos in JSON format. Once I have photos available I will simply show them on my page using some basic JQuery tricks.
To get things started, create an ASP.NET page and add JQuery library reference to the head section of the HTML page. Then add a DropDownList and a Button control on the page along with one simple span tag and a div tag with some CSS styles. Your page HTML markup should look like following:
<p>
Photos Category:
<asp:DropDownList ID="DropDownList1" runat="server">
<asp:ListItem Value="-1">Select</asp:ListItem>
<asp:ListItem Value="cat">Cats</asp:ListItem>
<asp:ListItem Value="bird">Birds</asp:ListItem>
<asp:ListItem Value="animal">Animals</asp:ListItem>
</asp:DropDownList>
<asp:Button ID="Button1" runat="server" Text="GO" />
<span id="progress" style="display: none;">Loading Images...</span>
</p>
<div id="thumbnails" style="display: none; width: 270px;
border: solid 2px #dadada; text-align: center; padding: 2px;">
</div>
From the above HTML code, you can easily guess that I am providing user some photo categories such as Cats, Birds and Animals to choose from in a DropDownList control. When the user will select the category and will click the GO button, I will run some JQuery code to fetch photos in the selected category from Flickr server. During the request, the span tag with the text “Loading Images…” will be displayed and once request processing will finish successfully I will add photos in the thumbnails div.
Next you need to add following JavaScript block with typical JQuery ready method in the head section of your page.
<script type="text/javascript" language="javascript">
$(document).ready(function ()
{
$("#Button1").click(function (e)
{
var category = $("#DropDownList1").val();
if (category != -1)
{
LoadPhotos(category);
}
e.preventDefault();
});
});
</script>
The above code looks very straight forward especially if you have read my previous tutorials on JQuery, or if you have some familiarity with JQuery code. In the above code, JQuery ready method defines the click event function handler for Button1 in which first the value of the selected photo category is retrieved and if the user has selectedany category such as Cats, Birds or Animals I am calling JavaScript function LoadPhotos, where all the magic will take place. Here is the implementation of the function LoadPhotos.
function LoadPhotos(category)
{
$("#progress").show();
var flickrUrl = "http://api.flickr.com/services/feeds/photos_public.gne?tags=" +
category + "&tagmode=any&format=json&jsoncallback=?";
$.getJSON(flickrUrl, function (data)
{
$("#thumbnails").empty();
$.each(data.items, function (i, item)
{
$("<img/>")
.css('margin', '3px')
.attr({ src: item.media.m, width: '60', height: '60', id: ('thumb' + i) })
.appendTo("#thumbnails");
});
$("#thumbnails").css('display', 'block');
$("#progress").hide();
});
}
First line of code is using simple JQuery selector to display the “Loading Images…” message, which is inside the span element with the id progress. Then I have declared a variable to store Flickr feed url, and you can see the selected category is concatenated inside the URL to provide the value for parameter tags.
$("#progress").show();
var flickrUrl = "http://api.flickr.com/services/feeds/photos_public.gne?tags=" +
category + "&tagmode=any&format=json&jsoncallback=?";
The most important line of code is the use of JQuery getJSON() method which loads JSON encoded data from the server using HTTP GET request. I have passed the Flickr URL as the first parameter of getJSON() function, and then I have used callback function to retrieve results and display images.
$.getJSON(flickrUrl, function (data)
{
...
});
Inside getJSON() callback function, I have used JQuery each function to loop through all the records available in the JSON response. Inside the JQuery each function, a new html <img> element is created for every photo. Its src has been set along with its height, width and id and in the end it is appended to the thumbnails div element.
$.each(data.items, function (i, item)
{
$("<img/>")
.css('margin', '3px')
.attr({ src: item.media.m, width: '60', height: '60', id: ('thumb' + i) })
.appendTo("#thumbnails");
});
The last two lines are to show the thumbnails div element on page and to hide the “Loading Images…” message.
$("#thumbnails").css('display', 'block');
$("#progress").hide();
That’s all you need to load and display photos from Flickr database on to your ASP.NET web page. You can take this simple example to any lever further and can create highly customizable photo gallery plug-ins, slide shows and widgets. If you will build and run your page you will see results similar to the figure shown in the start of this article.
You can download the full ASP.NET project source code used in this tutorial and can also watch the live demo in action by clicking the Live Demo button. Please keep visiting my website in the future for more useful tutorials and code samples.