In one of my previous article I have shown you how you can save images in database as binary data using File Upload control. I have received many requests to write an article on displaying binary images from the database in a data bound control such as DataList or GridView. In this tutorial I will show you how you can load images from database and display on ASP.NET DataList control.
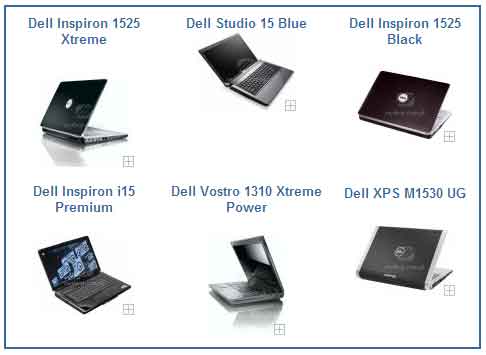
To complete this article, I assume that you have already stored images in database as binary data and if you are confused how to do this read my article Saving images in database using ASP.NET FileUpload Control. I saved some laptops with their images in my database for the purpose of this article.
I am using DataList control for this article but you can easily use same technique for Repeater or GridView control. First we need is to set up a simple DataList control that shows products from the database. Following is the HTML source for the DataList control.
<asp:DataList ID="DataList1" runat="server" RepeatColumns="3" RepeatDirection="Horizontal"
Width="100%" BorderColor="#336699" BorderStyle="Solid" BorderWidth="2px">
<ItemTemplate>
<asp:Label ID="Label1" runat="server" Text='<%# Eval("ProductName") %>' Font-Bold="True"
Font-Size="10pt" ForeColor="#336699" Width="100%" />
<br />
<asp:Image ID="Image1" runat="server"
ImageUrl='<%# "GetImage.aspx?id=" + Eval("ProductID") %>' />
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" VerticalAlign="Top" />
</asp:DataList>
The above code looks straight forward as it is using ItemTemplate of DataList control to display Product Name and Product Image. The interesting line for you is the Image control that has ImageUrl property set to another asp.net page which will actually generate images from the database.
You need to bind the above DataList control by using the code shown below. The code is simple ADO.NET code in which I fill one DataTable object and bind DataList control with the data table.
if (!Page.IsPostBack)
{
string constr = "Server=SampleServer; Database=SampleDB; uid=test; pwd=test";
string query = "SELECT ProductID, ProductName FROM Products";
SqlDataAdapter da = new SqlDataAdapter(query, constr);
DataTable table = new DataTable();
da.Fill(table);
DataList1.DataSource = table;
DataList1.DataBind();
}
Now we are moving to interesting part of this jigsaw puzzle, GetImage.aspx page. As I already told you that GetImage.aspx page main job is to read your binary image from the database and render it on the Image control. I am passing the Product ID to this page as query string as you have seen above in the Image control in the DataList ItemTemplate.
protected void Page_Load(object sender, EventArgs e)
{
string id = Request.QueryString["id"];
string constr = "Server=SampleServer; Database=SampleDB; uid=test; pwd=test";
string query = "SELECT ProductImage FROM Products WHERE ProductID = @ProductID";
SqlConnection con = new SqlConnection(constr);
SqlCommand com = new SqlCommand(query, con);
com.Parameters.Add("@ProductID", SqlDbType.Int).Value = Int32.Parse(id);
con.Open();
SqlDataReader r = com.ExecuteReader();
if (r.Read())
{
byte[] imgData = (byte[])r["ProductImage"];
Response.BinaryWrite(imgData);
}
con.Close();
}
In the above code I passed product id as parameter in the SELCT query to load particular product image from the database. The main trick is happening in the if condition where I retrieved binary image as byte array and write byte array to the response stream using BinaryWrite method of Response object.
I hope this article will help many others who thought that loading and displaying images in ASP.NET controls required lot of code.
Waqas,
Fantastic and Simple way to load… but just wondering what if we use ASHX rather than ASPX ??? Anyway this code was simply a nice one.
Thanks
How to make product slider of images retrieving from databaseusing datalist control
thanks 🙂 it worked !!
Hello sir ,
i have getting error for product id null show , how to get product id
thankyou
Hello, I’m getting an error when trying to assign the datatable to the datalist.
In here: DataList1.DataSource = table;
it’s returning:System.NullReferenceException: Object reference not set to an instance of an object.
Any ideas?
Thanks.
thanks alot.
We have to build 2 pages for this purpose ah…GetImage is one page nd we have to place DataList control in another page ah…plz help me,i m new to this concept…its very urgent for my project…reply me as soon as possible…
Can anyone tel me whr i have to give the Databind coding and whr i have to give the Page Load coding…Plz tel me clearly,its very urgent…
hi sir i need getimage.aspx page coding plese send me sir.
Hi sathyamoorthy, check Page_Load event above. It is what you need to put in getimage.aspx code behind file.
Your code is very usefull
I need help to change this code from C # to Visual Basic would help me a lot, please someone
Thanks,for code
Really this tutorial was useful thanks a lot but the image loads in static i need image to be loaded dynamically.
nice article
i think you also need to add Response.ContentType = “image/jpeg”
Thanks.
This worked!! Thank you! This was the easiest, most straight-forward way to get binary images off sql server and into datalist, or gridview or whatever you are using. When I understood that it sends value via querystring to the getimage.aspx page, It all made sense to me. Thanks again!
Hi your article was good….I Have a Query That Can We Retrieve Images From Different Tables And Display In Single Column Of Gridview ?
show only system byte[] on browser…
Exelent code………….
Your Code for display images in datalist is very useful for me.
A big THANX TO YOU
😀
Display images in DataList from Database in this article
in the place “string id = Request.QueryString[“id”]; ” the id values from where its always shows the null values ,and if “(!Page.IsPostBack)” code where i want to place
This site is very useful and all the examples given here are easily understood and they are straight forward to the solution.
I have small doubt I want to upload images from fileupload control and want to display in image control as soon as we upload. How to do that?
Great!!!, Simple!!!, Complete!!! and Well Explained (wihout story!!!). Which help me to understand and implement my task qucikly!
the page is not able to display any data, what might be wrong
Hi misteraddi
You must check your code by setting breakpoint. Check if you are getting data from database or not.
This is just amazing….. Thanks Lot
where we put up ado.net bind code
Hi Ramesh, Put the data binding code in Page_Load
hy how to show some of records in grid view.. i mean i want to display 3 records in grid view and when user click on show all button then all records will display.. can u help me??
Thank you, for this articel… it helps me great!!!
Torsten Lehmann
Berlin, Germany
i have do such of code but column are displayed but images are not visible still now,name are arranged in proper way but images are not displayed,what should i do i dont know ,please give some suggetion ,where i am doing mistake.
Thanks Sir,
your code for display images in datalist is very useful for me.
Thanks
Excelent, thnk you for this article
i want to upload images in data base
Respected Sir,
I want to perpare myself for MCTS Web Application but i dont know where i have to get start i have more than two year of development experience kindly if possible for you suggest me the road path for traveling.
Thanks
when i used this code the image is not displaying