In my previous post, Building GraphQL APIs in ASP.NET Core, we learned how to build a GraphQL server in ASP.NET Core using the Hot Chocolate library and Entity Framework Core and expose API endpoints to perform CRUD operations. In this post, I will show you how to consume the GraphQL APIs in the ASP.NET Core web application.
Table of Contents
Initial Project Setup
Open Visual Studio 2022 and create a blank solution with the name ConsumeGraphQLInAspNetCore. I will create the following two projects in this solution.
AspNetCoreGraphQLServer
The GraphQL Server project exposes the GraphQL API endpoints to be consumed by the client applications. I will not show you how to build the GraphQL server because I already covered this topic in greater detail in my previous posts Building GraphQL APIs in ASP.NET Core and Getting Started with GraphQL in ASP.NET Core. You can build a server by following these posts and make sure your server is up and running and you can see the GraphQL query results in the browser as follows:
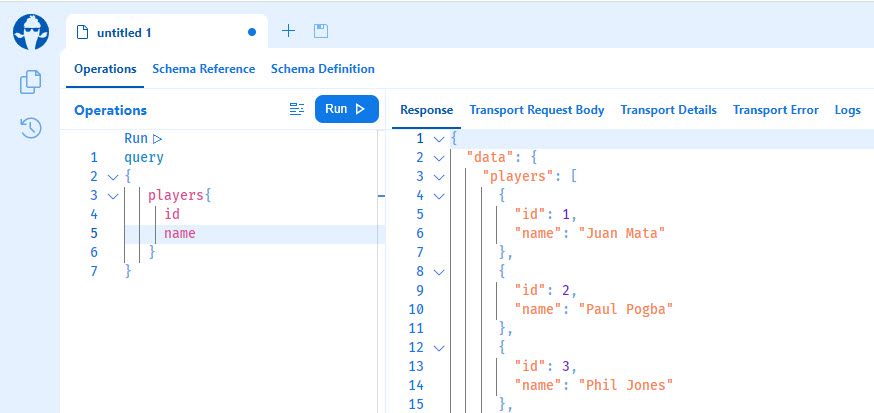
AspNetCoreGraphQLClient
This project will consume the APIs exposed by the GraphQL APIs exposed by the AspNetCoreGraphQLServer application. There are several GraphQL client libraries available for almost all popular programming languages but I will use the library called GraphQL.Client to simplify building GraphQL client application in C#.
Building GraphQL Client Application in ASP.NET Core
We are now ready to start building the GraphQL client application in ASP.NET Core. Before we start actual coding, we need to download and install the following libraries using the NuGet package manager.
- GraphQL.Client – Provides classes to build GraphQL. clients in .NET
- GraphQL.Client.Serializer.Newtonsoft – Provides serialization capabilities for GraphQL clients using Newtonsoft.Json as an underlying JSON library.
Next, we need to configure the GraphQL server endpoint in the appsettings.json file as follows:
appsettings.json
{
"GraphQLServerUri": "http://localhost:5043/graphql",
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*"
}
Next, we need to register GraphQLHttpClient with the ASP.NET Core dependency injection container in Program.cs file as follows. Notice, how we are reading the GraphQLServerUri from the appsettings.json file to initialize the EndPoint property of GraphQLHttpClientOptions.
Program.cs
builder.Services.AddScoped<IGraphQLClient>(s =>
new GraphQLHttpClient(new GraphQLHttpClientOptions()
{
EndPoint = new Uri(builder.Configuration["GraphQLServerUri"])
},
new NewtonsoftJsonSerializer()));
Once the GraphQLHttpClient is registered, we can inject it into any ASP.NET Core Controller or Razor Page and start writing GraphQL queries and mutations but I always like to keep my controllers and pages as lightweight as possible so I will create a separate service class that will contain GraphQL queries and mutations.
Implementing GraphQL Client Services
Create a Services folder in the AspNetCoreGraphQLClient project and add the following IPlayerService interface in the folder.
IPlayerService.cs
public interface IPlayerService
{
Task<IEnumerable<Player>> GetAllPlayersAsync();
}
Next, create a PlayerService class in the Services folder that will implement the above IPlayerService interface and will provide the implementation of the GetAllPlayersAsync method.
PlayerService.cs
public class PlayerService : IPlayerService
{
private readonly IGraphQLClient _client;
public PlayerService(IGraphQLClient client)
{
_client = client;
}
public async Task<IEnumerable<Player>> GetAllPlayersAsync()
{
var request = new GraphQLRequest
{
Query = @"
{
players {
id
name,
shirtNo,
appearances,
goals
}
}"
};
var response = await _client.SendQueryAsync<PlayersResponseType>(request);
return response.Data.Players;
}
}
In the above class:
We are injecting the IGraphQLClient object in the constructor and the ASP.NET Core dependency injection system will automatically inject the GraphQLHttpClient class object because we already registered this object in Program.cs file. GraphQLHttpClient has several methods that can be used to make API calls to GraphQL endpoints.
private readonly IGraphQLClient _client;
public PlayerService(IGraphQLClient client)
{
_client = client;
}
We are then creating an object of GraphQLRequest class and initializing its Query property with the string that represents the GraphQL query we want to execute from the client. We can specify the fields e.g. id, name, etc. as per client requirement.
var request = new GraphQLRequest
{
Query = @"
{
players {
id
name,
shirtNo,
appearances,
goals
}
}"
};
We are then calling the SendQueryAsync method that accepts a response type as a generic parameter. For our query, we passed the PlayersResponseType object which we will implement shortly.
var response = await _client.SendQueryAsync<PlayersResponseType>(request);
Finally, we are returning all the players we received from the server as an IEnumerable<Player> object.
return response.Data.Players;
We can now register our service in Program.cs file as follows:
builder.Services.AddScoped<IPlayerService, PlayerService>();
Creating Model Classes
In the above PlayerService class, we used some classes e.g. Player, PlayersResponseType, etc. Let’s implement those classes inside a Models folder of the project.
Player.cs
public partial class Player
{
public int Id { get; set; }
public int? ShirtNo { get; set; }
public string? Name { get; set; }
public int? PositionId { get; set; }
public int? Appearances { get; set; }
public int? Goals { get; set; }
}
PlayersResponseType.cs
public class PlayersResponseType
{
public List<Player> Players { get; set; }
}
Running GraphQL Queries from ASP.NET Core
Let’s create an ASP.NET Core Razor Page Index.cshtml to quickly test our PlayerService class. The page code behind class will inject IPlayerService through the constructor and will call the GetAllPlayersAsync method of the PlayserService class to initialize a local Players property.
Index.cshtml.cs
public class IndexModel : PageModel
{
private readonly IPlayerService _playerService;
public IEnumerable<Player>? Players { get; set; }
public IndexModel(IPlayerService playerService)
{
_playerService = playerService;
}
public async Task<IActionResult> OnGetAsync()
{
Players = await _playerService.GetAllPlayersAsync();
return Page();
}
}
The razor view will contain a simple HTML table that will iterate over the Players and display the player’s information received from the GraphQL server endpoint.
Index.cshtml
@page
@model IndexModel
@{
ViewData["Title"] = "Players";
}
<div>
<h3>Players List</h3>
<br />
<table class="table">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Shirt No</th>
<th>Appearances</th>
<th>Goals</th>
</tr>
</thead>
<tbody>
@if (Model.Players != null)
{
foreach (var player in Model.Players)
{
<tr>
<td> @player.Id </td>
<td> @player.Name </td>
<td> @player.ShirtNo </td>
<td> @player.Appearances </td>
<td> @player.Goals </td>
</tr>
}
}
</tbody>
</table>
</div>
Before you run both client and server applications, make sure both projects are set as the startup project in Visual Studio. This is important because we want our GraphQL server application up and running when the GraphQL client application is making API calls. For this task, you need to open Set Startup Projects… dialog from the Project menu.
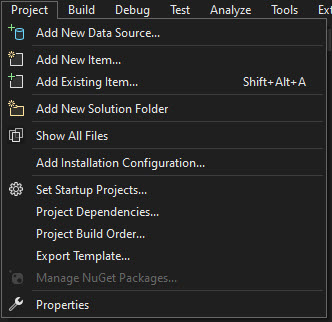
In the dialog, you need to select both AspNetCoreGraphQLServer and AspNetCoreGraphQLClient projects in the Multiple startup projects section.
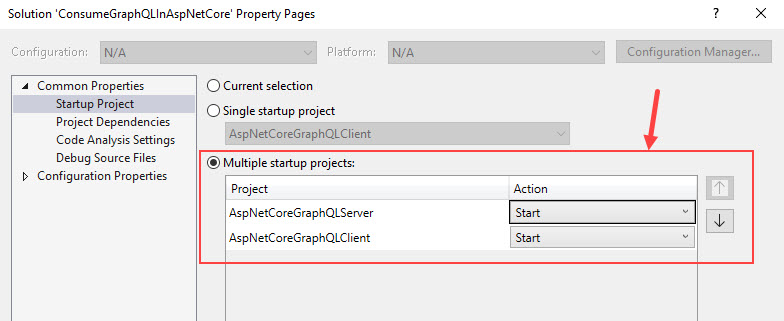
Press F5 to run both server and client applications in parallel and you should see the client application showing the player’s information as shown in the screenshot below.
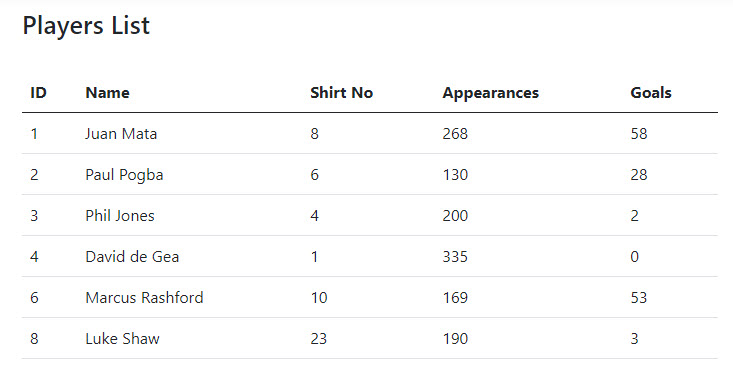
Summary
In this post, we learned how to prepare a GraphQL client application in ASP.NET Core application using GraphQL.Client library and how to consume GraphQL APIs exposed by the servers. The complete source code including both AspNetCoreGraphQLServer and AspNetCoreGraphQLClient projects is available on GitHub so you can download and play with the code. If you have any comments or suggestions, please leave your comments below. Don’t forget to share this tutorial with your friends or community.
Hi , Thanx for tutorial. It would be great if you help in authentication part
Hi
Thanks for the tutorial, it was very helpful, I only have one question if the GraphQL server requires requestHeader to authenticate to the service, how would it be done?