In one of my previous tutorials I have shown you how you can transform XML documents using XSLT files with the help of ASP.NET XML server control. I have received many queries asking me how we can achieve similar functionality programmatically. In this tutorial I will show you how to transform an XML document using .NET Framework built in XmlCompiledTransform class available in System.Xml.Xsl.
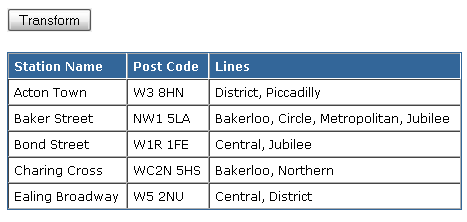
XmlCompiledTransform class acts as the XSLT processor in .NET Framework 2.0 and used to transform XML data into other formats. Please keep in mind that you should not use XmlTransform class of .NET Framework 1.x for XSL transformations in your applications because is now obsolete and is replaced with new XmlCompiledTransform class. According to MSDN, the new XmlCompiledTransform class provides huge performance improvements over its predecessor.
For the purpose of this tutorial, I am using following XML file:
TubeStations.xml
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<undergroundList>
<underground>
<name>Acton Town</name>
<lines> District, Piccadilly</lines>
<postCode>W3 8HN</postCode>
</underground>
<underground>
<name>Baker Street</name>
<lines> Bakerloo, Circle, Metropolitan, Jubilee</lines>
<postCode>NW1 5LA</postCode>
</underground>
<underground>
<name>Bond Street</name>
<lines> Central, Jubilee</lines>
<postCode>W1R 1FE</postCode>
</underground>
<underground>
<name>Charing Cross</name>
<lines> Bakerloo, Northern</lines>
<postCode>WC2N 5HS</postCode>
</underground>
<underground>
<name>Ealing Broadway</name>
<lines> Central, District</lines>
<postCode>W5 2NU</postCode>
</underground>
</undergroundList>
I am using following XSLT file to render XML data in HTML format. You are free to use any XML or XSLT file in your projects.
GridViewStyle.xslt
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output method="html" indent="yes"/>
<xsl:template match="/undergroundList">
<div style="font-family:Verdana; font-size:9pt;">
<table style="width:100%; border: solid 1px #336699;" cellspacing="0"
cellpadding="5" border="1">
<tr style="background-color:#336699; color:white; font-weight:bold;">
<td>Station Name</td>
<td>Post Code</td>
<td>Lines</td>
</tr>
<xsl:for-each select="underground">
<tr>
<td><xsl:value-of select="name"/></td>
<td><xsl:value-of select="postCode"/></td>
<td><xsl:value-of select="lines"/></td>
</tr>
</xsl:for-each>
</table>
</div>
</xsl:template>
</xsl:stylesheet>
Now you have both XML and XSLT files and you are ready to write some code to programmatically transform the above XML document using the XSLT file. For demonstration I am creating a simple ASP.NET page which only has one Button and Literal controls on it. As you can guess I will write all the code in the Button Click event.
First I am declaring two string variables to store the path of both XML and XSLT files.
string xmlFile = Server.MapPath(".") + "\\TubeStations.xml";
string xslFile = Server.MapPath(".") + "\\GridViewStyle.xslt";
Next I am creating object of XmlCompiledTransform class and Loading XSLT file using its Load method. Keep in mind that you need to import the reference of System.Xml.Xsl namespace to use XmlCompiledTransform class.
XslCompiledTransform processor = new XslCompiledTransform();
processor.Load(xslFile);
The Transform method of XmlCompiledTransform class transforms the XML file and place output into a stream so I am creating MemoryStream object to store the generated output.
MemoryStream ms = new MemoryStream();
Once everything is ready I am transforming the XML file by using Transform method. This method has many overloads and the one I am using here needs XML file URI, optional arguments and the output stream to write the generated output.
processor.Transform(xmlFile, null, ms);
The remaining code is creating a StreamReader object to read all the generated contents from the memory stream as string so that I can display the contents on Label or Literal control.
ms.Seek(0, SeekOrigin.Begin);
StreamReader reader = new StreamReader(ms);
string output = reader.ReadToEnd();
ms.Close();
Literal1.Text = output;
I hope now you have basic idea how XmlCompiledTransform class works and you can take this basic tutorial to next step and can explore more XML and XSLT related classes available in .NET Framework.