The visitors to a web site can typically be divided into two groups: the users and the administrators. In data driven web sites, users are normally allowed to view data and administrators are allowed to add, edit or delete data. In this tutorial I will show you how you can create an editable GridView control for administrators to modify database records directly into the GridView control.
Editing the GridView control can be an incredibly easy feature to add for certain database tables, especially those who have no foreign key associated and can easily be edited through the use of a standard TextBox web control as shown in the figure below:
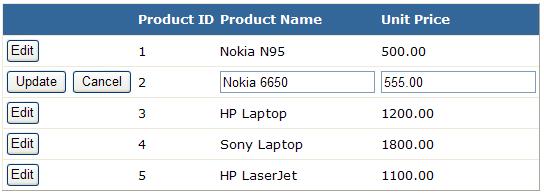
Before we start examining the ASP.NET GridView markup and associated code lets first enumerate the important code pieces of this tutorial.
- You need to include the GridView CommandField column to add Edit, Update and Cancel Buttons in the GridView.
- You need to include TemplateField for every column you want to provide Editing because TemplateField provides you both ItemTemplate and EditItemTemplate to create interface for two separate GridView modes.
- You need to handle GridView events such as RowEditing, RowCancelingEdit and RowUpdating to provide desired editing functionality.
Following is the HTML content for an ASP.NET GridView that provides editing support for the database table.
<asp:GridView ID="GridView1" runat="server" CellPadding="3" GridLines="Horizontal"
Font-Names="Verdana" Font-Size="10" DataKeyNames="ProductID"
AutoGenerateColumns="false"
onrowcancelingedit="GridView1_RowCancelingEdit"
onrowediting="GridView1_RowEditing" onrowupdating="GridView1_RowUpdating">
<HeaderStyle BackColor="#336699" ForeColor="White" HorizontalAlign="Left" Height="25" />
<Columns>
<asp:CommandField ButtonType="Button" ShowEditButton="true" ShowCancelButton="true" />
<asp:BoundField DataField="ProductID" HeaderText="Product ID" ReadOnly="true" />
<asp:TemplateField HeaderText="Product Name">
<ItemTemplate>
<%# Eval("ProductName")%>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox runat="server" ID="txtProductName" Text='<%# Eval("ProductName")%>' />
</EditItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Unit Price">
<ItemTemplate>
<%# Eval("UnitPrice")%>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox runat="server" ID="txtUnitPrice" Text='<%# Eval("UnitPrice")%>' />
</EditItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
In the above code, I set two properties ShowEditButton and ShowCancelButton of CommandField. These properties will automatically render Edit, Update and Cancel buttons inside GridView column. I am using TemplateField’s ItemTemplate and EditItemTemplate for providing read only and editing interface of the same column in the GridView. By Default, GridView control displays ItemTemplate which directly shows the ProductName and UnitPrice columns but when user clicks Edit button GridView automatically switches to EditItemTemplate and display the control available inside EditItemTemplate for user editing.
Now I will show you the C# code behind file of the above ASP.NET page that will handle different GridView events to provide complete editing functionality.
In the Page_Load event, you simply load your data from the database and bind it with the GridView control as shown below:
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
BindData();
}
}
private void BindData()
{
string constr = @"Server=COMPAQ\SQLEXPRESS; Database=TestDB; uid=waqas; pwd=sql;";
string query = "SELECT ProductID, ProductName, UnitPrice FROM Products";
SqlDataAdapter da = new SqlDataAdapter(query, constr);
DataTable table = new DataTable();
da.Fill(table);
GridView1.DataSource = table;
GridView1.DataBind();
}
For brevity I am not storing connection string in the web.config and also not using any Data Access Component.
Next event is the GridView RowEditing event to give ASP.NET GridView index no of the editable row. You need to set the EditIndex property of the GridView with the NewEditIndex property of the GridViewEditEventArgs class.
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
BindData();
}
If user clicks the Cancel button while GridView is in editing mode, you need to Cancel the Editing process and also need to reset the EditIndex property to -1 in the RowCancelingEdit event of the GridView control as shown below:
protected void GridView1_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
e.Cancel = true;
GridView1.EditIndex = -1;
BindData ();
}
The last step is to handle the RowUpdating event to actually update the back end database. To do this we first need to obtain the reference of the current row by using its index no as shown in the first line below. By using the GridViewRow object we can find the reference of any control in the row in which user is editing the data. In this tutorial we are searching for our TextBox controls txtProductName and txtUnitPrice. To get the Primary Key reference we can use the DataKeys collection of the GridView. This collection only requires the row index no and it will give us the primary key. Last line of the following code is calling a local method for updating the database and passing all the parameters to this method.
protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
GridViewRow row = GridView1.Rows[e.RowIndex];
TextBox txtProductName = (TextBox)row.FindControl("txtProductName");
TextBox txtUnitPrice = (TextBox)row.FindControl("txtUnitPrice");
int productID = Int32.Parse(GridView1.DataKeys[e.RowIndex].Value.ToString());
string productName = txtProductName.Text;
decimal unitPrice = Decimal.Parse(txtUnitPrice.Text);
UpdateProduct(productID, productName, unitPrice);
}
Following code is the UpdateProduct method that is using standard ADO.NET Connection and Command objects to run Update query in the database.
private void UpdateProduct(int productID, string productName, decimal unitPrice)
{
try
{
string constr = @"Server=COMPAQ\SQLEXPRESS; Database=TestDB; uid=waqas; pwd=sql;";
string query = "UPDATE Products SET ProductName = @ProductName, UnitPrice = @UnitPrice WHERE ProductID = @ProductID";
SqlConnection con = new SqlConnection(constr);
SqlCommand com = new SqlCommand(query, con);
com.Parameters.Add("@ProductName", SqlDbType.NVarChar).Value = productName;
com.Parameters.Add("@UnitPrice", SqlDbType.Decimal).Value = unitPrice;
com.Parameters.Add("@ProductID", SqlDbType.Int).Value = productID;
con.Open();
com.ExecuteNonQuery();
con.Close();
GridView1.EditIndex = -1;
BindData();
}
catch (Exception ex)
{
throw ex;
}
}
Please keep in mind that there are many different ways to update the database using the GridView control depending on the type of data you are updating and the functionality the user need and there are many solutions to achieve the same end result. In the above tutorial I tried to give you one of the easiest solutions and I hope you can go explore GridView control editing further after learning the basics from this tutorial.
Nice example, it´s work!!
thanks a lot , was very usefull to me!!!
I tried but got an error like missed reference value
Thank you for writing this, after searching thru an unknown number of web sites, your tutorial had my issue resolved within 10 min! 6 years later this information is still relevant and helps others resolve issues. Can’t thank you enough!
hi i am getting “Object reference not set to an instance of an object” error after update row, it’s like run all th grid wiew twice
Hi object reference not set to instance object means u wrote connection under the try block that’s why it showing write the connection out side the try block then it work fine
thanks…
Thanks
great. finally got what i was looking for.thanks
Hello ,
Best Article from all the Live websites…seriously very simple and explained everything…super Like…!!!!
THanks Bhia
Normally in gridivew, All buttons are enabled (Edit, Update or Cancel)
Is it possible to force the user to Click on Update/Cancel button, when in Record Edit Mode. ? and then to Click on Edit for other rows.
Thanks
Thanks..for the article…Nice one
Nice Post…Very Good for Begineers…
A very good and most helpful for .net users
venku .plz make all other fields as Readonly(true) .
This is an excellent tutorial really easy to understand quickly .good work
This is an excellent tutorial really easy to understand quickly .good work
Even though I made only one column as template filed, the entire row is displaying as a editable ? why all the columns are showing in edit mode ?
This is mostly helpful to any .net user
This article is very useful for which save a day for me
thanks a lot
very good article about data grid view. is there any method to format manually data grid view in asp.net.
a very good and useful article indeed
thank you
thanxx….
its very nice to learn for beginers
hi i am getting “Object reference not set to an instance of an object” error…
pls any one let me know why it comes
Set breakpoint to check which line is causing “Object Reference not set” error.
I have tried ut code of Updating Row of gridview but it coudn’t find latest value from controls, and grdiview is not updating.
how to do multiple records editing / updating with checkbox?
Thanks