ASP.NET AJAX UpdatePanel control allows you to divide your page into self-contained regions which can be updated asynchronously without a full page postback. However, this control assumes that the user must initiate the asynchronous request either by clicking a button or by using any other control. In some situations you might want to perform partial or full page updates without waiting for a user action such as stock or news tickers or dynamically updatable advertisements. In this tutorial I will show you how you can use ASP.NET AJAX Timer control to initiate asynchronous request and update your page contents automatically.
AJAX Timer control enables a portion of an ASP.NET web page to be dynamically updated at a regular interval without the need of a user perform an action. Please keep in mind that excessive use of Time controls can reduce the scalability of your application and you should use long intervals rather than many small intervals which will increase overhead of your application.
It is very straightforward control and has very few properties to set. It has Interval property to specify the number of milliseconds which should be elapsed before an update. Following markup shows the use of AJAX Timer control with UpdatePanel to display different book title and image after every 2 seconds.
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<br />
<asp:UpdatePanel ID="UpdatePanel1" runat="server" UpdateMode="Conditional">
<ContentTemplate>
<asp:Label ID="Label1" runat="server" Font-Size="14pt">AJAX in Action</asp:Label>
<br />
<br />
<asp:Image ID="Image1" runat="server" ImageUrl="~/images/ajax_in_action.jpg" />
</ContentTemplate>
<Triggers>
<asp:AsyncPostBackTrigger ControlID="Timer1" EventName="Tick" />
</Triggers>
</asp:UpdatePanel>
<asp:Timer ID="Timer1" runat="server" Interval="2000" OnTick="Timer1_Tick">
</asp:Timer>
In the above markup, please notice that the UpdateMode property of the UpdatePanel is set to Conditional and I am also using Triggers collection of UpdatePanel control to initiate the asynchronous request. You should use this approach when you are using Timer control and you should not simple place your Timer control inside the UpdatePanel as it can cause a full page postback.
The Timer control raises a server side Tick event which you can handle to update your page contents with any application specific logic. In this tutorial I am just updating the book title and image on the page every time the Tick event fires.
protected void Timer1_Tick(object sender, EventArgs e)
{
NameValueCollection list = new NameValueCollection();
list.Add("AJAX in Action", "images/ajax_in_action.jpg");
list.Add("AJAX Bible", "images/ajax_bible.jpg");
list.Add("Understanding AJAX", "images/understanding_ajax.jpg");
Random r = new Random();
int index = r.Next(0, 3);
Label1.Text = list.Keys[index].ToString();
Image1.ImageUrl = list[index].ToString();
}
If you will run this tutorial you will see one of the followings book title and image will be displayed on page after every two seconds.
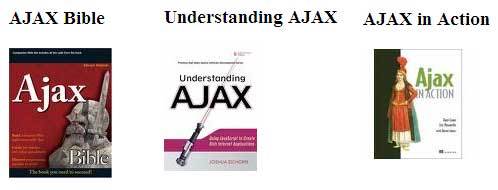
this code does not work says index is out of range
Hi Anant
Please make sure you are not getting random no between 0 to 2 to make this code work.