When you perform AJAX bases asynchronous postback using ASP.NET UpdatePanel control it performs its job in the background and the user keep working with the other page elements and controls. This is generally what you want to for better user experience but sometimes you have an asynchronous request that takes little longer and you want to show user some visual indication that the request is being processed and the update is under way. In this tutorial I will show you how you can use UpdateProgress control available in ASP.NET AJAX server side controls gallery to simulate the progress indicators.
The UpdateProgress control works in conjunction with the UpdatePanel control and allows you to show a text message or an animated GIF while a time-consuming update is in progress. This control can be added any where on the page and is not need to be added inside UpdatePanel control. When you add the UpdateProgress control to a page, you provide its contents in ProgressTemplate either at design time or in HTML source view. These contents will appear as soon as an asynchronous request is started and disappear as soon as request is finished.
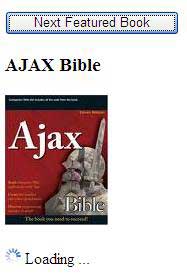
The markup below shows you the use of UpdateProgress control in conjunction with UpdatePanel control:
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<br />
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<asp:Button ID="Button1" runat="server" Text="Next Featured Book"
OnClick="Button1_Click" />
<br />
<br />
<asp:Label ID="Label1" runat="server" Font-Size="14pt">AJAX in Action</asp:Label>
<br />
<br />
<asp:Image ID="Image1" runat="server" ImageUrl="~/images/ajax_in_action.jpg" />
</ContentTemplate>
</asp:UpdatePanel>
<br />
<asp:UpdateProgress ID="UpdateProgress1" runat="server">
<ProgressTemplate>
<img alt="" src="images/progress.gif" /> Loading ...
</ProgressTemplate>
</asp:UpdateProgress>
The button click event handler code below is choosing one random book title along with its image from the NameValueCollection and for making sure this request take little longer I have added Thread.Sleep() method with 2 seconds sleep time.
protected void Button1_Click(object sender, EventArgs e)
{
System.Threading.Thread.Sleep(2000);
NameValueCollection list = new NameValueCollection();
list.Add("AJAX in Action", "images/ajax_in_action.jpg");
list.Add("AJAX Bible", "images/ajax_bible.jpg");
list.Add("Understanding AJAX", "images/understanding_ajax.jpg");
Random r = new Random();
int index = r.Next(0, 3);
Label1.Text = list.Keys[index].ToString();
Image1.ImageUrl = list[index].ToString();
}
The UpdateProgress control has DisplayAfter property that takes number of milliseconds and decide how quickly you want ProgressTemplate to appear inside UpdateProgress control. It also has AssociatedUpdatePanelID property to associate the UpdateProgress control with a particular UpdatePanel control on the page. Once it is associated it will only work asynchronous postback of associated UpdatePanel control.
The following markup demonstrates how you use multiple UpdateProgress control with AssociatedUpdatePanelID property set to different UpdatePanel control.
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<br />
<table class="style1">
<tr>
<td>
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<asp:Button ID="Button1" runat="server" Text="Show Server Time"
OnClick="Button1_Click" />
<asp:Label ID="Label1" runat="server"></asp:Label>
</ContentTemplate>
</asp:UpdatePanel>
</td>
<td>
<asp:UpdatePanel ID="UpdatePanel2" runat="server">
<ContentTemplate>
<asp:Button ID="Button2" runat="server" Text="Show Server Time"
OnClick="Button2_Click" />
<asp:Label ID="Label2" runat="server"></asp:Label>
</ContentTemplate>
</asp:UpdatePanel>
</td>
</tr>
<tr>
<td>
<asp:UpdateProgress ID="UpdateProgress1" runat="server"
AssociatedUpdatePanelID="UpdatePanel1">
<ProgressTemplate>
<img alt="" src="images/progress.gif" /> Updating ...
</ProgressTemplate>
</asp:UpdateProgress>
</td>
<td>
<asp:UpdateProgress ID="UpdateProgress2" runat="server"
AssociatedUpdatePanelID="UpdatePanel2">
<ProgressTemplate>
<img alt="" src="images/progress.gif" /> Updating ...
</ProgressTemplate>
</asp:UpdateProgress>
</td>
</tr>
</table>
The code behind file with Button Click events is as follows:
protected void Button1_Click(object sender, EventArgs e)
{
System.Threading.Thread.Sleep(2000);
Label1.Text = DateTime.Now.ToString("hh:mm:ss tt");
}
protected void Button2_Click(object sender, EventArgs e)
{
System.Threading.Thread.Sleep(2000);
Label2.Text = DateTime.Now.ToString("hh:mm:ss tt");
}
Following figure shows the output of the above markup.
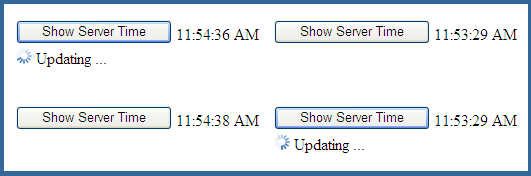
Good One 🙂