The ObjectDataSource control allows you to bind your data bound controls to custom .NET business objects Using ObjectDataSource control, accessing and displaying data from a Business Login Layer can be accomplished without writing a single line of code. In this tutorial I will show you how you can use ObjectDataSource control to call methods from custom business objects and how you can bind ObjectDataSource control with ASP.NET data bound controls such as GridView.
The ObjectDataSource Control
The ObjectDataSource control allows you to connect your data bound controls with your Business Logic Layer objects to produce the output. Like SqlDataSource control this control also supports declarative parameters to allow developers to pass variables or controls values to business objects as parameters. When ObjectDataSource is configured to call methods from a custom .NET class it makes some assumptions about that class. The class should have a default constructor and should have methods which can easily be mapped to select, update, insert or delete operations.
The ObjectDataSource control provides almost the same programming interface as the SqlDataSource control. It has nearly same methods, properties and events with the addition of some new events and properties. It has properties such as SelectMethod, UpdateMethod, InsertMethod and DeleteMethod with corresponding parameters collections such as SelectParameters, UpdateParameters, InsertParameters, and DeleteParameters to perform typical database operations. It also has TypeName property that returns the fully qualified name of the class you want to use to call methods.
To see ObjectDataSource control in action, I am starting this tutorial with one simple example in which I will connect ObjectDataSource control with a custom class that will return the List collection of a custom Book objects. Following is the code of the Book and BookManager classes
Book.cs
public class Book
{
public int ID { get; set; }
public string Title { get; set; }
public double Price { get; set; }
public Book(int id, string title, double price)
{
this.ID = id;
this.Title = title;
this.Price = price;
}
}
BookManager.cs
public class BookManager
{
public List GetBooks()
{
List list = new List();
list.Add(new Book(101, "Java", 34.99));
list.Add(new Book(102, "HTML", 31.99));
list.Add(new Book(103, "XSLT", 37.99));
list.Add(new Book(104, "XML", 59.99));
list.Add(new Book(105, "XHTML", 35.99));
list.Add(new Book(106, "CSS", 35.99));
return list;
}
}
You can see in the above code snippet that I will use BookManager class with ObjectDataSource control and will call GetBooks method to display all books in GridView control. Next you need to configure ObjectDataSource control on the page by setting its TypeName and SelectMethod properties as following code shows:
<asp:ObjectDataSource ID="ObjectDataSource1" runat="server"
SelectMethod="GetBooks" TypeName="BookManager" />
Once your ObjectDataSource is configured you can associate it with any data bound control with the help of DataSourceID property as shown in the following GridView code snippet.
<asp:GridView ID="GridView1" runat="server" DataSourceID="ObjectDataSource1"
BorderColor="#336699" BorderStyle="Solid" BorderWidth="2px" CellPadding="4"
Font-Names="Verdana" Font-Size="10pt">
<HeaderStyle BackColor="#336699" ForeColor="White" />
</asp:GridView>
If you will run this program you will see the following output on the page.
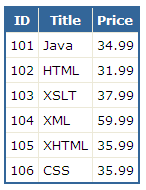
In the example above, the GetBooks method returns a List collection filled with custom Book objects but you can also return other type of objects such as collections which have interface IEnumerable implemented a DataReader, a DataTable or even DataSet.
To demonstrate this, I have created another business object named SqlHelper with two methods which are returning DataReader and DataSet objects:
public class SqlHelper
{
public SqlDataReader GetProductsDataReader()
{
string constr = "Server=TestServer; Database=SampleDatabase; uid=test; pwd=test;";
string query = "SELECT ProductID, ProductName, UnitPrice FROM Products";
SqlConnection con = new SqlConnection(constr);
SqlCommand com = new SqlCommand(query, con);
con.Open();
return com.ExecuteReader(CommandBehavior.CloseConnection);
}
public DataSet GetProductsDataSet()
{
string constr = "Server=TestServer; Database=SampleDatabase; uid=test; pwd=test;";
string query "SELECT ProductID, ProductName, UnitPrice FROM Products";
SqlConnection con = new SqlConnection(constr);
SqlCommand com = new SqlCommand(query, con);
SqlDataAdapter da = new SqlDataAdapter(com);
DataSet ds = new DataSet();
da.Fill(ds);
return ds;
}
}
You can configure ObjectDataSource control to use the above methods with a similar two properties you used above in the Books List example:
<asp:ObjectDataSource ID="ObjectDataSource1" runat="server"
SelectMethod="GetProductsDataReader" TypeName="SqlHelper" />
<asp:ObjectDataSource ID="ObjectDataSource2" runat="server"
SelectMethod="GetProductsDataSet" TypeName="SqlHelper" />
In the end you can bind any of the above control with your databound control as shown below:
<asp:GridView ID="GridView1" runat="server" DataSourceID="ObjectDataSource1"
BorderColor="#336699" BorderStyle="Solid" BorderWidth="2px" CellPadding="4"
Font-Names="Verdana" Font-Size="10pt">
<HeaderStyle BackColor="#336699" ForeColor="White" />
</asp:GridView>
<asp:GridView ID="GridView1" runat="server" DataSourceID="ObjectDataSource2"
BorderColor="#336699" BorderStyle="Solid" BorderWidth="2px" CellPadding="4"
Font-Names="Verdana" Font-Size="10pt">
<HeaderStyle BackColor="#336699" ForeColor="White" />
</asp:GridView>
Both of the above GridView controls will produce the following output on your page.
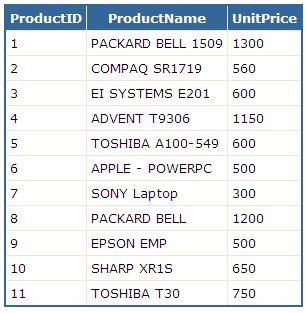
I hope now you have basic understanding of ObjectDataSource control and you will start using it more and more in your own projects specially if you have layered application architecture and you want to call methods from Business Logic or Data Access Layer.
Wonderful tutorial! Thank you!
Dude u ROCK!!! I spent many hours on video tutorials to understand object data source. Within 5 min. i learned it with ur tutorial.
Thank you very much !!!
I had not worked with this object before., I wanted to know how it worked and this is the best documentation I found, clear, precise and very well explained.
bye.
Thank you
this article is helpful and i was search for it
thanks again
This is what you call clean and simplified tutorial.Thanks for sharing.God bless