In one of my other article “Using Checkbox in ASP.NET GridView Control”, I have shown you how you can update the row status in database using checkboxes in GridView. There are situations when you want to provide checkboxes in GridView for deleting multiple database records similar to Hotmail or Yahoo inboxes. GridView control allows you to delete only a single record at a time but in this tutorial I will show you how you can implement multiple records deletion scenario in GridView control.
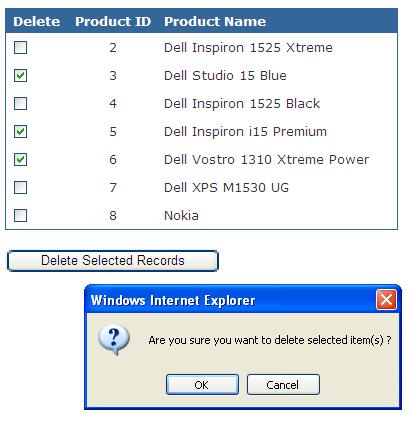
To start this tutorial you add the GridView and Button controls on ASP.NET page and make sure your code looks similar to following:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" Width="40%">
<Columns>
<asp:TemplateField HeaderText="Delete">
<ItemTemplate>
<asp:CheckBox runat="server" ID="chkDelete" />
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="ProductID" HeaderText="Product ID">
<HeaderStyle HorizontalAlign="Center" />
<ItemStyle HorizontalAlign="Center" />
</asp:BoundField>
<asp:BoundField DataField="ProductName" HeaderText="Product Name">
<HeaderStyle HorizontalAlign="Left" />
<ItemStyle HorizontalAlign="Left" />
</asp:BoundField>
</Columns>
</asp:GridView>
<br />
<asp:Button ID="btnDelete" runat="server" OnClick="btnDelete_Click" Text="Delete Selected Records" />
The code above is straight forward and the important thing is the use of TemplateField to add a CheckBox control in every row of the GridView control. The data is provided to GridView control in the Page_Load event as follows:
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
BindData();
btnDelete.Attributes.Add("onclick",
"return confirm('Are you sure you want to delete selected item(s) ?');");
}
}
private void BindData()
{
string constr = @"Server=TestServer; Database=SampleDB; uid=test; pwd=test;";
string query = @"SELECT * FROM Products";
SqlDataAdapter da = new SqlDataAdapter(query, constr);
DataTable table = new DataTable();
da.Fill(table);
GridView1.DataSource = table;
GridView1.DataBind();
}
Notice how I added Delete confirmation to the button using the Attributes collection in the Page_Load event. The rest of the code is binding the GridView to a Products table using the SqlDataAdapter and DataTable classes available in ADO.NET. If you will test your page you will see GridView with checkboxes similar to the figure shown above in the start of the tutorial. Now you have to write code on the Click event of the Button in which you need to check which products are selected for deletion. Following is the code for Delete button click event:
protected void btnDelete_Click(object sender, EventArgs e)
{
ArrayList productsToDelete = new ArrayList();
foreach (GridViewRow row in GridView1.Rows)
{
if (row.RowType == DataControlRowType.DataRow)
{
CheckBox chkDelete = (CheckBox)row.Cells[0].FindControl("chkDelete");
if (chkDelete != null)
{
if (chkDelete.Checked)
{
string productId = row.Cells[1].Text;
productsToDelete.Add(productId);
}
}
}
}
DeleteProducts(productsToDelete);
BindData();
}
In the above code I first created an ArrayList to store product id for all the selected products used has selected to delete. Then I am running a simple foreach loop to iterate all the rows in the GridView. Remember we are only interested in the rows where we have checkboxes we are not interested in Header, Footer or Pager rows that’s why I put one if condition check in the loop to make sure we only process data rows. Then I am getting the reference of every single CheckBox in the GridView using Cells collection and FindControl method. After I have Checkbox control available I am checking whether it is checked or not using Checked property and if it is checked I am reading Product ID of that product in second cell in the same row. I am also storing the product id in the productsToDelete ArrayList for later processing. Once the foreach loop is finished I called a custom method DeleteProducts and passed the productsToDelete ArrayList to the method. I am not implementing the code for DeleteProducts method but you can implement it easily once you know which items are available to delete from the database. The declaration of DeleteProducts will be similar to following:
private void DeleteProducts(ArrayList productsToDelete)
{
// Execute SQL DELETE Command to Delete all Products
// available in productsToDelete collection
// You can also pass productsToDelete ArrayList to
// your Data Access Layer component for delete operation
}
I hope you will be able to implement this scenario in your own ASP.NET website easily without facing any big issue.
the row btnDelete.Attributes.Add( …);
should be outside/above the if (!Page.IsPostBack)
>> otherwise the confirm button only appears the first time …
it is very helpful for me. pls provide me the delete part
plzzzz also define
private void DeleteProducts(ArrayList productsToDelete)
{
}
plzzzz
good code!!!!
Nice code sir… It helped a lot…Thank You 🙂
I would like to use gridview with a checkbox to append data to a table
Can you please post the syntax for the SQL DELETE command?
please send delete code
private void DeleteProducts(ArrayList productsToDelete){
}
Helpful article and very easy to implement.
Thanks,
i ve used the same code but every time i m getting the null value of checkbox
really helpful
can u give brief idea in 3 tier architecture plzzzzzzzzzzzzzzzzzzzzzzzz
hey can u explain the last part with some piece of code
exelent code thanks……….
dfsdf
i want coding to delete selected checkbox
i am verry verry satisfy………
But how to delete if, gridview is enabled with paging option?
Hi,This is nice code,i will thank full for you.And can you send the code of private void DeleteProducts(ArrayList productsToDelete) method.please
fantastic answer!!!!!!
exelent code thanks
Sir ur articles are ver gud for beginners.. Keep up the gud work..Thanks a lot.. If possible plzz provide the source code for all the articles… Thankz
waqas bhai very good example .Helped me a lot . If possible can u mail me the delete code part which u have left in this example, as i m new to dotnet i m not able to do it.
thank you
please provide me code file for deleting multiple records from gridview .
thanks for sharing! Appreciated~~
can u give delete procedure also…
Thanks 4 the cool soln…. 🙂
nice code
Good Code
i like it……
Thank for your intruction
Can you write detail DeleteProducts method ? I still dont understand.
Thank for your intruction
Can you write detail DeleteProducts method ? I still dont understand.
can you please provide me code for inserting rows into sql in grid view using checkbox
Thank You for Your Code
Hello , i want to use short cut key of keyboard for deletion of records. can anyone help me?
You can use java script to attach short cut keys with buttons. I also
remember there is property to attach short cut keys with ASP.NET buttons.
I think its AccessKey property.
Very Good Code
good code
i am satisfy