I hope you have probably heard about AJAX by now, or at least you have used an AJAX based web application such as Gmail or Yahoo Mail, etc. AJAX has changed the way we developed web sites in the past by giving them responsiveness similar to Desktop applications. In Plain English, AJAX means that instead of waiting for the whole web page to load you can load and display only what you need to. Technically speaking, it’s a technique to communicate with servers through JavaScript asynchronously and handle external data. In this tutorial, I will show you how you can call ASP.NET web service using JQuery, which is the most popular and widely used JavaScript library.
To start communicating with ASP.NET web service through JQuery we first need to implement our web service. Create an ASP.NET web site project in Visual Studio and add an ASP.NET Web Service by right clicking the project name and choosing Add New Item option as shown in the figure below.
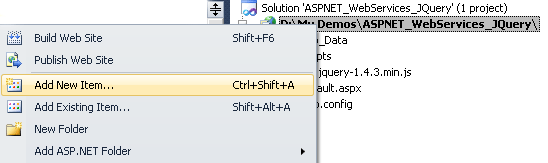
Once the Add New Item dialog box appears, select Web Service (.asmx) item from the list and give web service a suitable name such as DateWebService.asmx. Visual Studio will create a web service (.asmx) and related code behind files in the project. You need to add a method GetDateTime in web service code behind file but keep in mind that we will call this web service from JavaScript so you need to remove comments from the attribute System.Web.Script.Services.ScriptService of your class. The complete code of the web service class is shown below.
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class DateWebService : System.Web.Services.WebService {
public DateWebService () {
//Uncomment the following line if using designed components
//InitializeComponent();
}
[WebMethod]
public string GetDateTime() {
return DateTime.Now.ToString();
}
}
The web service code is pretty straight forward as there is only one method GetDateTime defined returning the current Date and Time using DateTime.Now property. Before calling the service method using JQuery you need to keep in mind few restrictions on calling service methods from JavaScript. To preventing some security vulnerabilities, ASP.NET service validation layer checks for following two things when service methods are called from JavaScript.
- Only POST method can be used to call a web service method. You cannot use HTTP GET.
- The HTTP content-type header should be set to the value “application/json”.
For those of you who are not familiar with JSON may be wondering what is json and where it came from. So don’t worry I have some basic introduction for you in this tutorial as we will use it in few minutes using JQuery AJAX. In modern AJAX based web applications, when web services are called from client applications, they normally return XML formatted response, which can be parsed and use in JavaScript, but it complicates things for developers. They need a simple data structure which can be used more easily than XML. This is where JavaScript Object Notation (JSON) comes to help us with the following major features.
- Lightweight data-interchange format.
- Easy for humans to read and write.
- Easy for machines to parse and generate.
- JSON can be parsed trivially using the JavaScript eval() procedure or using some useful methods in JQuery API.
- JSON supports almost all modern languages such as ActionScript, C, C#, ColdFusion, Java, JavaScript, Perl, PHP, Python, Ruby and many more.
Any .NET object you return from web service methods can be serialized in JSON format. To get some familiarity with JSON format, let’s say you have following custom Product object initialized with some properties.
Product product = new Product();
product.Name = "Apple";
product.Expiry = new DateTime(2008, 12, 28);
product.Price = 3.99M;
product.Sizes = new string[] { "Small", "Medium", "Large" };
If you will serialize the object in JSON format it will produce the following output:
{
"Name": "Apple",
"Expiry": new Date(1230422400000),
"Price": 3.99,
"Sizes": [
"Small",
"Medium",
"Large"
]
}
You can see from the above output that JSON data structure is very easy to read and understand. If you are a .NET developer and want to learn more about serialization and de-serialization of .NET objects into JSON, I will recommend you to use free JSON.NET library available at json.codeplex.com. Let’s come back to our tutorial, we have implemented web service so it’s time to create a web page and call that service using JQuery. Create an ASP.NET web form (.aspx) in your project and add the following button and span tag in web form.
<form id="form1" runat="server">
<input id="Button1" type="button" value="Get Date and Time" />
<br />
<br />
<span id="output" />
</form>
Next add the JQuery library reference and the following JQuery script in the head section of the page.
<script src="Scripts/jquery-1.4.3.min.js" type="text/javascript"></script>
<script type="text/javascript">
$(document).ready(function(){
$("#Button1").click(function() {
$.ajax({
type: "POST",
url: "/ASPNET_WebServices_JQuery/DateWebService.asmx/GetDateTime",
data: "{}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (msg)
{
$("#output").text(msg.d);
}
});
});
});
</script>
The important lines in the above code are when the $.ajax() method is called inside the Button1 click event handler. $.ajax() method performs an asynchronous HTTP AJAX request and can be customized with many parameters. Some of the parameters I used in the above code are explained below:
type: The type of request you want to make “POST” or “GET”. The default value of this parameter is “GET”
url: A string containing the URL to which the request is sent. In the code above, I put the website project name followed by the service file name with extension and then the web method name.
data: This parameter contains the data to be sent to the server. For the GET requests it will be appended to the url although you can change this behavior using processData parameter (not used in the above example). I am using empty braces in the above example as I am not passing any parameters to service methods.
contentType: When sending data to the server, use this content-type. Default is “application/x-www-form-urlencoded”. Data will always be transmitted to the server using UTF-8 charset. In the above code, I am explicitly using application/json as the contentType.
dataType: The type of data that you’re expecting back from the server. If none is specified, JQuery will intelligently try to get the results, based on the MIME type of the response. The available types are xml, html, script, json, jsonp and text.
success: JQuery $.ajax() method also supports many callback functions, which are invoked at the appropriate during the request process. One of them is a success which is called if the request succeeds.
In this tutorial, I have explained all the options used in this example code above but there are more parameters and options available, and If you are interested to read more about JQuery $.ajax() method please visit the JQuery API documentation available at http://api.jquery.com/jQuery.ajax.
Build and test your page in the browser, and you will see the server time displayed on the page when you will click the button. If you are using FireFox you can even see the JSON formatted response came from the server in my favorite FireFox plug-in Firebug.
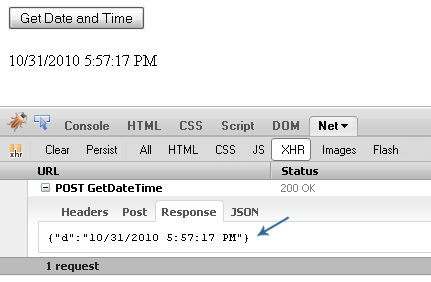
In this tutorial, I tried to give you the basic introduction of calling ASP.NET web service using JQuery AJAX along with some explanation of JSON format. In my future tutorials, I will show you more complex interaction between client and server using JQuery AJAX so please keep visiting my website to learn more about the tips and tricks of latest technologies.
Hey! Thanks a lot!
You saved my day =)
Hi, I need someone that could help me, because I tried the downloaded example and it didn’t work.
I opened it in VS 2013, when I try the DataWebService.asmx, it returns me the date, but when is called from the Default.aspx, nothing happened.
Could you help me?
Thanks in advance.
Its so simple and understandable Example, Thank you so much.
I am new for this technology but from your example, i learn properly.
Thanks man this helped a lot.
Thanks Very helpful. But i want instead of webservice from local project i need external webservice calling in Ajax JQuery JSON.
issue resolved. I put the wrong ajax url.
Thanks for your great article.
Hi Waqas:
Big thanks for your details about asp.net web service and JQuery ajax script.
However, I tried my asp.net web project from VS2010 but it is not working after I clicked the button.(Jquery button click event was called but no ajax action)
Do you know what going on is?
Sincerelys,
Nice article with step by step instructions. Easy for understanding. Thank you 🙂
your article is very simple yet vry explainatory.Its vry useful for new users.
Thanks alot 🙂
This is an explanatory and clean example on the topic from the rest of the search.
nice explanation and loved it the way u conveyed
thanks
Dear,
Your article was very simple and very very useful. I am using this chance to say deeeeeep thanks to you.
Thank you ….
Nice explanation of calling web Service.
Azhar Iqbal
Dot not 65
The article is nice, but could be better if you show people how to pass parameters as well.
thanks
very helpful
Very Nice tutorial