JQuery is one of the most common libraries used in web development today and it has been used very frequently by ASP.NET MVC developers for not only enhance the user experience but also to provide dynamic contents to their web application users using different type of AJAX calls supported by JQuery. JQuery provides several methods such as get, getJSON, load, post, ajax which can be used to call different types of ASP.NET MVC controller’s actions. In this tutorial, I will show you how you can use JQuery get method to call ASP.NET MVC actions.
Table of Contents
Getting Started
Using jQuery with the ASP.NET MVC is very easy because its latest version ships with ASP.NET MVC. To use jQuery you just have to include the following script tag either in the <head> section of the Layout.master page or at the bottom before the closing </body> element for faster page loading.
<script src="<%= Url.Content("~/Scripts/jquery-1.3.2.js") %>" type="text/javascript"></script>
This will load the jQuery library from the content folder and make it available on the page.
Scenario 1: Calling an action method that returns string content
Let’s assume that you have an action that returns string content to views as follows:
public class HomeController : Controller
{
public string GetDateTimeString()
{
return DateTime.Now.ToString();
}
}
To call this action method using jQuery, you can use the jQuery ‘get’ function as shown below:
var url = "/Home/GetDateTimeString";
$.get(url, null, function(response) {
$("#getDateTimeString").html(response);
});
The jQuery ‘get’ method is a helper method that generates an AJAX GET request. The first parameter is the URL of the action method, the second parameter can be used to pass additional parameters to the action method and the third is the callback function needed to be called when the response is received from the action method. The callback function takes one parameter ‘’response’ that holds the string content. The following is the output of the resulting fetched from server:
6/4/2015 12:38:41 PM
Scenario 2: Calling an action method that takes parameters and returns string content
This is almost the same scenario as the previous one, except that this time the action method expects a parameter in request. To pass the action method parameters we can use the second parameter of the jQuery ‘get’ function.
public string LowerCaseString(string input)
{
return input.ToLower();
}
You can use the following JavaScript code to call this action method from JQuery get method:
var url = "/Home/LowerCaseString";
$.get(url, { input: "MAKE THIS TEXT LOWERCASE" }, function(response) {
$("#getLowerCaseString").html(response);
});
make this text lowercase
Scenario 3: Calling an action method that takes parameters and returns JSON
So far we are playing with simple strings, Now imagine you want to dynamically fetch more complex data from server and the data is formatted in JSON. For example, imagine we want to fetch student information from the server. More specifically let’s say you have the following StudentInfo class and GetStudentInfo action method:
public class StudentInfo
{
public string FirstName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
public int Fee { get; set; }
}
public JsonResult GetStudentInfo(int studentId)
{
StudentInfo contactInfo = new StudentInfo
{
FirstName = "Bob",
LastName = "Cravens",
Fee = 2000,
Age = 23
};
return Json(contactInfo, JsonRequestBehavior.AllowGet);
}
Notice the action method is now returning a ‘JsonResult’. This action method can be called using the following JavaScript:
var url = "/Home/GetStudentInfo";
var id = 123;
$.getJSON(url, { studentId: id }, function(data) {
$("#name").html(data.FirstName + " " + data.LastName);
$("#fee").html(data.Fee);
$("#age").html(data.Age);
});
You can view the AJAX call response coming in JSON using the browser developer tools. For example, the Google Chrome browser is showing the following JSON coming from server.
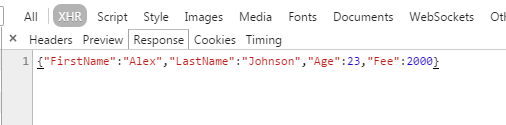
Notice the response data now formatted in JSON. The returned data can be placed inside any html element such as span as shown below:
<h3>Student Information</h3>
<table>
<tr>
<td>Name</td>
<td><span id="name"></span></td>
</tr>
<tr>
<td>Fee</td>
<td><span id="fee"></span></td>
</tr>
<tr>
<td>Age</td>
<td><span id="age"></span></td>
</tr>
</table>
The following will be the output:
Student Information
Name Alex Johnson
Fee 2000
Age 23
hi,
I am working asp.net MVC.
and I want to call actions of controller of MVC with angularjs.
actions like: create-select-delete-edit-update
Can you help me?
i also want same
thanks, good article.