This is my first tutorial on Android, so I have decided to start with a simple example. Android ListView control is one of the most popular controls available to Android developers, and It can be used in many different forms and can be customized to suit almost every possible application requirement. In this tutorial, I will demonstrate you how you can bind a simple array of Strings with ListView. In my future tutorials, I will show you more advance scenarios and layouts that can be achieved using ListView.
Before, I start, I am assuming that you have installed Eclipse, configured your Android development environment along with ADT, and you know how to create and run a simple Android project.
Create a new Android project in Eclipse with MainActivity as your default Activity and main.xml as your default layout file. Declare a ListView control in your main.xml layout file as shown in the following code.
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<ListView
android:id="@+id/listView1"
android:layout_height="fill_parent"
android:layout_width="fill_parent" />
</LinearLayout>
The above code is using simple LinearLayout with vertical orientation, and a ListView is declared to cover the entire width and height of the parent container using the fill_parent as the value of both android:layout_height and android:layout:width properties. ListView also has a unique id listView1 that will be used in the MainActivity to reference the ListView control.
Following is the code you need in MainActivity.java. The code first access the ListView control by passing the generated id to findViewById function. Next the array of Strings is declared with some simple list of items in it. Next an instance of ArrayAdapter object is created by passing it the reference of current activity context using this. A reference of the Android predefined TextView resource id along with the array of objects as parameters in the constructor. In the end, adapter is provided to ListView using setAdapter function available in ListView class.
MainActivity.java
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
ListView listView1 = (ListView) findViewById(R.id.listView1);
String[] items = { "Milk", "Butter", "Yogurt", "Toothpaste", "Ice Cream" };
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1, items);
listView1.setAdapter(adapter);
}
}
Run the project in Eclipse and you will see the output similar to the following screenshot in the Emulator.
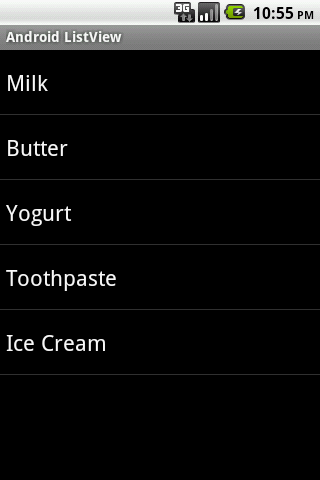
thank you so much for this simple easy to understand illustration.. very straightforward.
how to webapi name ,and username, get the value in list view in api
I’ve found a easier way :
<listview android:id=”@+id/nav_list” android:entries=”@array/nav_drawer_items” android:layout_width=”match_parent” android:layout_height=”match_parent” android:layout_below=”@+id/nav_top”/>
the “android:entries” fills automatically the listView with the array.
I am using SOAP web services calling Soap method GetVehicle and showing it in Textview as an Json array then Save the data in Sqlite database Table Vehicle , now I want to populate Name column of the Vehicle table from SQLite database into ListView.
I have searched a lot of examples but all are showin an user defined array items in listview, but not any example which is using Name column of Table from sqlite into ListView.
Plz do help me by contributing in my code or any sample code r examples.
I am biggner for android ,it is very usefull to me ,thanks
this tutorial made updating the arrayadapter easy…
facing problem at this point
list.setAdapter(adapter);
Thanks for tutorial. What if one want to add images and check boxes in the list view. Please upload this tutorial too.
android.R.layout.simple_list_item_1 show eror
simple and efficient =)
That is very nice example.
thank you dude
ASSALAMALAIKUM!!
sir here you have declared items of the listview on main activity page,but what if i have an array containing data fetched from any url…..now i have bind that data in ListView,any suggestions??
Thanks for Such a nice and simple tutorial
I am a newbee to android. and it is so helpful for me. Thanks for shared your knwldge..
How would I make the height of each row in the ListView… to about 1/2 as tall?
how to configure Android development environment along with ADT ???
please also write tutorial.
how to configure android project…
from beginning..