If you are developing .NET applications from some time, you may already know how to connect databases and performs operations using either hard coded SQL queries or stored procedures. You may also know how to query the in memory collection of objects using traditional programming constructs such as loops and conditions. In this tutorial, I will give you introduction to Microsoft’s new technology called LINQ that enables you to integrate query processing features into your programming language such as C# or Visual Basic. More specifically I will show you how you can query in memory object collections or Arrays using some basic LINQ query operators.
What is LINQ?
Language Integrated Query (LINQ) provides .NET developers some new and exciting features to query and manipulate data in a consistent and uniform way. It enables them to work with different type of data sources such as Arrays, collection of objects, XML documents, a relational databases, Active Directory or even Microsoft Excel sheets by writing or embedding queries in C# or Visual Basic language.
Microsoft has introduced many new keywords and features in both C# and Visual Basic languages to integrate LINQ technology. Microsoft has also added new namespaces in .NET Framework Library that contains new interfaces and hundreds of extension methods also called LINQ query operators. These methods perform different operations on different type of data sources in LINQ.
The following figure shows you the overall architecture of LINQ building blocks.
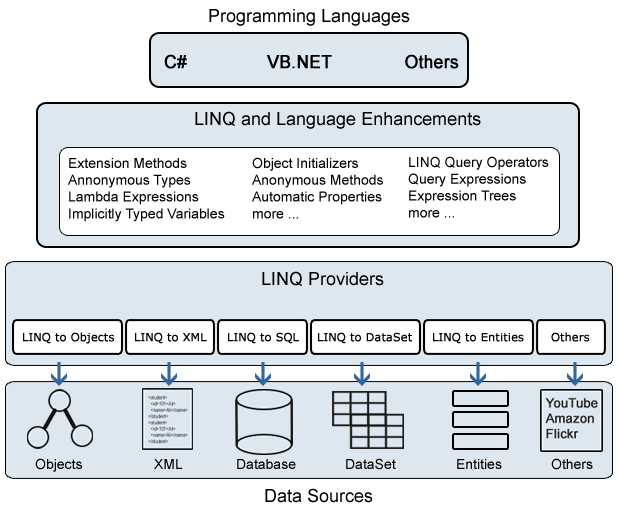
In the above architecture you can see that many new features are introduced in both C# and Visual Basic to integrate LINQ queries into language. You can also notice how different type of data sources can be accessed using LINQ providers and related language enhancements in a unified way.
One common question that comes in everybody’s mind is that if we are already using almost all the above data sources in the applications we are created then why we need to use LINQ. The answer of this question is that you need to learn different object models and technologies to connect all the above data sources. For example, if you want to connect relational databases you need to learn ADO.NET data provider object such as SqlConnection, SqlCommand, SqlDataReader along with the knowledge of SQL language. Similarly if you want to manipulate XML documents you should have knowledge of DOM or Simple API for XML (SAX).
To give you some idea about how you can write simple LINQ queries I am giving you a simple LINQ example in C# in the following code. I am creating a simple array of strings which I will query using some new C# keywords and standard LINQ query operators.
string[] monthNames = {
"January", "February", "March", "April",
"May", "June", "July", "August", "September",
"October", "November", "December"
};
I will query the above array of strings in such a way that I only get month names which are shorter than 6 letters. Here is the query that will perform this task:
var shortNames = from name in monthNames
where name.Length < 6
select name;
In the above query, Notice how the new keywords such as from, where and select are used. They look similar to the keywords used in SQL language and they provide the basis for writing LINQ queries. The order of the query looks rather reverse and it may feel strange to you if you have SQL query syntax in mind.
The first keyword from determines the data source used to query and select information in this example it is an array of strings. In LINQ world, the data source is called sequence. The variable name is declared just after the keyword from to hold the current enumerated value temporarily. This temporary variable is used in the second line in the above code alone with another new keyword where that performs filtering. Most of the LINQ queries you will write require some sort of filtering on the data so you can use where keyword to filter data source depending on the needs of your application. Finally I am using the select keyword to actually select the value I want to include in the query results. Notice the results of the query are stored in an implicitly typed variable shortNames which is defined using var keyword.
To display the results of the query you can use simple foreach loop:
foreach (var item in shortNames)
{
Console.WriteLine(item);
}
If you will compile and run the above foreach loop, here is the output you’d see:
March
April
May
June
July
Let’s say you also want to display data in specific order, You can add another new operator orderby in the above query to perform this task as shown below:
var shortNames = from name in monthNames
where name.Length < 6
orderby name.Length
select name;
The orderby keyword changes the order of the data according to your own criteria. In the example here I have ordered the months names according to their length so the shortest month name will come on top of the results. Here is the output you will get after running the above query.
May
June
July
March
April
So far, the queries I wrote look very straight forward and I haven’t introduced you another important building block of LINQ technology called query operators.
What are LINQ Query Operators?
LINQ Query operators are not a language extension, but an extension to the .NET Framework Class Library. These are a set of extension methods to perform operations in the context of LINQ queries. These operators are at the heart of the LINQ foundation and they are the real elements that make LINQ possible. It is not possible to cover all the query operators in one tutorial because there are hundreds of them but I will give you some easy examples of some operators which we have already used above without even knowing that they are operators. For example, when we use select, where and orderby keywords above we were actually using query operators. These query operators are extension methods available in many new namespaces added in .NET Framework to facilitate LINQ technology.
The following is the list of standard query operators along with their category:
Category | Operators |
---|---|
Projection | Select, SelectMany |
Filtering | OffType, Where |
Sorting | OrderBy, OrderByDescending, ThenBy, ThenByDescending, Reverse |
Set | Distinct, Except, Intersect, Union |
Concatenation | Concat |
Aggregation | Aggregate, Average, Count, LongCount, Max, Min, Sum |
Element | ElemenatAt, ElementAtOrDefault, First, FirstOrDefault, Last, LastOrDefault, Single, SingleOrDefault |
Conversion | AsEnumerable, AsQuerable, Cast, OfType, ToArray, ToDictionary, ToList, ToLookup |
Equality | SequenceEqual |
Generation | DefaultifEmpty, Empty, Range, Repeat |
Grouping | GroupBy, ToLookup |
Join | Join, GroupJoin |
Quantifiers | All, Any, Contains |
Partitioning | Skip, SkipWhite, Take, TakeWhite |
The code below shows you how you can map the keywords you have used in above query examples to standard query operators. I am using same example I have used above to give you better comparison of operators and their use.
var shortNames = monthNames
.Where(name => name.Length < 6)
.OrderBy( name => name.Length)
.Select(name => name);
The output of the above query will be similar to the above queries.
May
June
July
March
April
Notice how I have used three query operators Where, OrderBy and Select in the above syntax. Also notice the use of lambda expressions inside the methods. Some C# developers found this query operator syntax easier to understand than the above mentioned syntax because it is calling simple methods from the classes available in .NET Framework. Every method performs its operation on the query result it gets and then passes the results to another extension method. Almost all query operators return the object of type IEnumerable<T> which is a sequence of objects and can be queried further by using another operator. For example, you can perform the above three operations using three separate C# statements using one query operator at a time.
var shortNames = monthNames.Where(name => name.Length < 6);
var shortNamesWithOrder = shortNames.OrderBy( name => name.Length);
var finalResults = shortNamesWithOrder.Select(name => name);
Now if you can query the finalResults variable using foreach loop
foreach (var item in finalResults)
{
Console.WriteLine(item);
}
In this tutorial, I tried my best to give you a very basic introduction of LINQ with simple explanation and examples. LINQ is a big topic and there are hundreds of books in the market now covering the technology in detail. I will recommend you to keep visiting my website to read new tutorials on LINQ in future.
Was really helpful, was struggling with this for a week,,,Thanks. Jeny
really useful materials thanks i dont know about linq here only going to learn so please send basic of linq
really help full ….thanx
Very Nice tutorial….thank you very much.
There is a typing error, please correct it :-
“var finalResults = shortNames.Select(name => name);”
In this last line , you have missed type “shortNames.Select…..” There should “shortNamesWithOrder.Select….”
its very helpfull sir thanks!!!