Windows Communication Foundation (WCF) is a framework designed for software and web developers who want to develop distributed service-oriented applications. WCF allows developers to create, host, consume and secure services using Microsoft platform in a most productive way so that developers can focus on their implementation rather than communication protocols and low level messaging details. In this tutorial, I will cover the basic concepts needed to work with WCF services. You will be able to create and consume services using the most commonly used features of WCF technology.
Table of Contents
Introduction
Modern applications no longer work stand alone, they communicate with devices, client software and other services. Microsoft Office, SharePoint, iPod, XBOX 360, AJAX, RSS, Gaming and 3D Graphics environments are some examples of such applications which combine locally installed software with remote web services. Some of these applications use REST protocol over HTTP to ensure simple, cacheable, stateless, client server communication while others use SOAP protocol to ensure more robust, reliable and secure way for exchanging complex data. WCF allows developer to implement services in a unified way regardless of the wire protocol or message format used to communicate. WCF combines .NET Remoting, Distributed Transactions, Message Queues and Web Services into a single programming model and also support interoperability with other processes. The following figure shows the different technologies available in WCF framework.
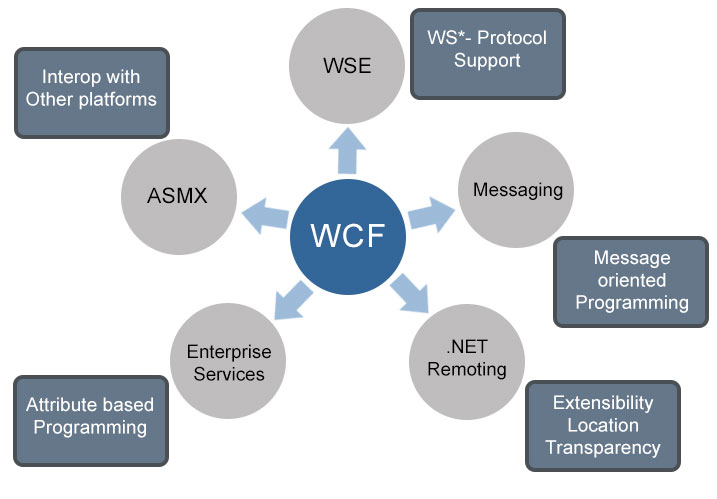
Features of WCF
Following section list the key features of Windows Communication Foundation (WCF).
Service Orientation
One of the features of using WCF is that it allows you to build service-oriented applications. These applications use services to send and receive data and provide the benefit of loose-coupling. In a loosely-coupled service-oriented architecture any client can connect to hosted services as long as the client meets the service contract requirements.
Interoperability
WCF supports interoperability with multiple web services specifications and implements a number of web services protocols. WCF provides support for Web services (WS) infrastructure protocols through channels and Web services application protocols through the contracts feature. Interoperability for application protocols is accomplished through XML Schema description language 1.0 (XSD) and Web Services Description Language (WSDL) 1.1. Some of the major protocols/specifications include HTTP 1.1, SOAP 1.1, SOAP 1.2 Core, XML, WSDL 1.1, XOP etc.
Multiple Message Patterns
WCF supports multiple patterns for exchanging messages from one endpoint to other endpoint. The most common pattern is request/reply pattern, where one endpoint sends data request to second endpoint and second endpoint replies to the request. In one-way message pattern, first endpoint sends a message to second endpoints and do not expect the reply from the second endpoint. Duplex exchange pattern is also supported where two endpoints establish a connection and send data back and forth just like an instant messaging program.
Service Metadata
WCF supports service metadata publishing using industry standards formats such as WSDL, XML Schema and WS-Policy. This metadata can be published over HTTP or HTTPs and client applications use this metadata to generate or configure service clients who can communicate with the WCF services.
Data Contracts
Data Contracts are .NET classes to represent a data entity with properties that belong to the data entity. These classes are used to exchange data between WCF services and clients. WCF service architecture automatically generates the metadata from these classes that allows clients to send and receive data.
Security
WCF supports messages encryption to protect data privacy using well-known standards such as SSL or WS-SecureConversation. It also supports authentication to prevent unauthorized access to services from users or client applications.
Multiple Transports and Encodings
WCF supports several built-in transport protocols and encodings for messages communication. On the World Wide Web, messages are text encoded in SOAP format and send over HTTP. Other supported options are using TCP, Named pipes or MSMQ. Furthermore, messages can be encoded as text or optimized binary format which can be send efficiently using the MTOM standard. WCF also allows you to create your own custom transport or encoding if none of the built-in option suits your need.
Transactions
WCF supports three different transaction models. You can use WS-AtomixTransactions, the APIs in the System.Transactions namespace and Microsoft Distributed Transaction Coordinator.
AJAX and REST Support
To support the modern Web 2.0 technologies such as REST, JSON, ATOM etc. WCF can be configured to process the data in plain XML format without wrapping it in SOAP envelope.
Extensibility
If you are running short of options or existing built-in features doesn’t fulfill your needs, WCF provides you many extensibility entry points to customize the behavior of a service according to your application requirements.
Key Terms and Concepts
WCF system introduces some new terms whose understanding is important to be more productive in working with services.
Endpoints
Each WCF service exposes one or more endpoints to its clients and all the communication takes place through those endpoints. In simple words, an endpoint is a resource on the network to which clients can send messages. These messages are formatted according to the contract on which both client and service are agreed. For successful and meaningful communication between client and the service both parties needs to know the Address of the service, the binding and the contracts commonly known as ABCs of WCF.
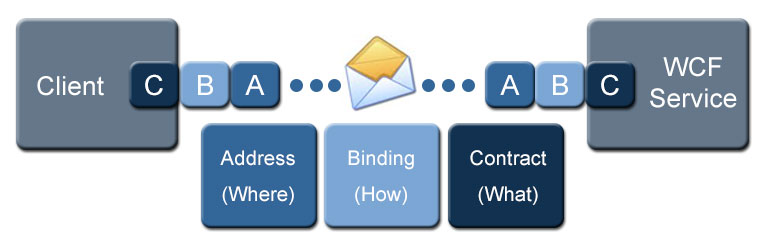
Address
The address (where) defines the location to which the messages must be send by the client to start the communication with the WCF service. For HTTP, the address would look like http://webserver/webservice and for TCP, it would look like net.tcp://webserver:8080/webservice.
Binding
The binding (how) specifies how client will communicate with the service. It defines a serious of binding elements which can be configured to suit your application needs. The lowest level binding element is the transport that defines the base protocol to be used such as HTTP, Named Pipes, TCP, MSMQ etc. Encoding element defines the type of encoding to be used such as Text, Binary or MTOM. Other elements are related to security, transaction or reliable messaging capability etc.
Fortunately, WCF has some predefined bindings with all these binding elements correctly configured for you to save your time of figuring it out yourself. Some of the most common bindings are shown below:
Binding | Description |
---|---|
BasicHttpBinding | Basic Web service communication. No security by default |
WSHttpBinding | Web services with WS-* support. Supports transactions |
WSDualHttpBinding | Web services with duplex contract and transaction support |
NetMsmqBinding | Communication between WCF applications by using queuing. Supports transactions |
NetNamedPipeBinding | Communication between WCF applications on same computer. Supports duplex contracts and transactions |
NetPeerTcpBinding | Communication between computers across peer-to-peer services. Supports duplex contracts |
NetTcpBinding | Communication between WCF applications across computers. Supports duplex contracts and transactions |
Contract
The contract (what) is an agreement between two or more parties for common understanding and it is a platform-neutral and standard way of describing what the service does. In WCF, contracts are mapped to interfaces which describe the list of operations exposed to the clients from a particular endpoint. The following three types of contracts are supported by WCF.
Operation Contract: Defines the signature and the list of parameters to be passed in and out of those operations.
[OperationContract]
double AddNumbers(double a, double b);
Service Contract: Defines the service that holds multiple operation contracts and act as a single functional unit.
[ServiceContract]
public interface ICalculator
{
[OperationContract]
double AddNumbers(double a, double b);
[OperationContract]
double DivideNumbers(double a, double b);
[OperationContract]
void FormatNumbers(double a);
}
Data Contract: Defines the external format of the data passed to and from the service operations. It defines the structure and types of data exchanged and can map the CLR type to an XML Schema. It can also define how the data will be serialized over a network.
[DataContract]
public class ComplexNumber
{
[DataMember] public int RealNumber { get; set; }
[DataMember] public int BaseNumber { get; set; }
}
A WCF service can expose multiple endpoints where each endpoint is defined by an address, binding and contract. Clients can also implicitly host endpoints to facilitate the message flow which is typically bidirectional.
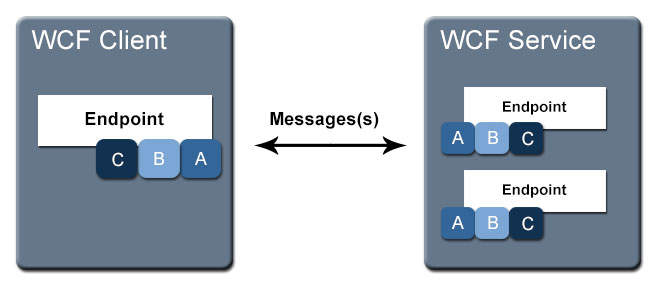
WCF Architecture
The figure below shows the major layers of Windows Communication Foundation (WCF) architecture.
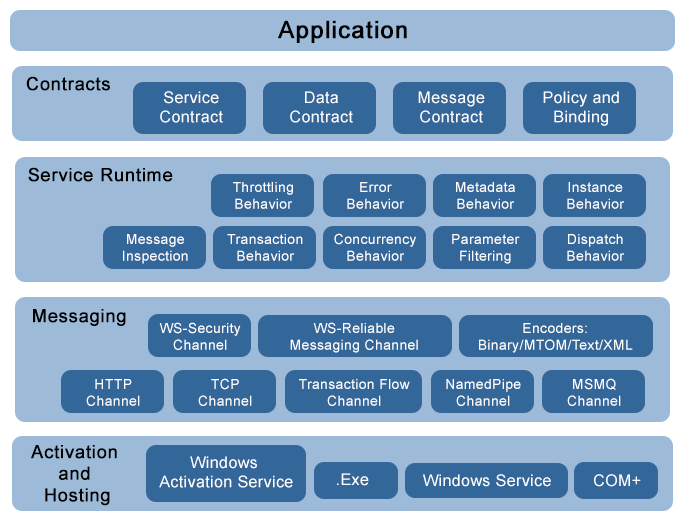
Contracts
Contracts layer define various aspects of the WCF messaging system. The data contract describes the data to be passed between the service and client and the way to serialize that data. The message contract defines the default SOAP message format provided by the WCF runtime for communication between client and service and it can be customized to meet your requirements. The service contract specifies the actual method signatures of the service and usually defined as an interface. Policy and bindings define the conditions required to communicate with a service such as transport protocol HTTP or TCP, an encoding, security requirement etc.
Service Runtime
The service runtime layer contains the runtime behaviors of the service that occur only during the actual operation of the service.
- Throttling Behavior – Controls how many messages are processed and can be configured if the demand of the service increased.
- Error Behavior – Specifies what occurs when an internal error occurs on the service. This allows you to configure what information is communicated to the client when any exception occurs.
- Metadata Behavior – Tells how and whether metadata is available to outside world.
- Instance Behavior – Specified how many instance of the service has to be created while running. For example, a singleton specified only one instance to process all messages.
- Transaction Behavior – Enables the rollback of transacted operations if a failure occurs.
- Dispatched Behavior – Controls how a message is processed by the WCF infrastructure.
Messaging
The messaging layer is composed of channels. A channel is a component that processes a message in some way such as by authenticating a message. Channels usually operate on messages and message headers and this is different form the service runtime layer which is mainly concerned about processing the contents of message bodies. Two types of channels are supported: Transport channels read and write messages from the network. Examples are HTTP, TCP, MSMQ, Named pipes etc. Protocol channels implement message processing protocols by reading or writing additional headers to the message. Examples of these protocols include WS-Security and WS-Reliability.
Activation and Hosting
All WCF Services must be hosted before they start listening to client requests. A WCF service can be hosted in following ways:
- IIS – Internet Information Service provides number of advantages if a service used HTTP as protocol. IIS hosting option is integrated with ASP.NET and used the features these technologies offer such as process recycling, idle shutdown, process health monitoring and message-based activation. This is preferred solution for hosting web service applications that must be highly available and highly scalable.
- Windows Activation Service (WAS) – WAS is the new process activation mechanism that ships with IIS 7.0 and available for the Windows Server 2008 or Windows 7. In addition to HTTP based communication, WCF can also use WAS to provide message-based activation over other protocols, such as TCP and named pipes.
- Self-Hosting – WCF service can be self-hosted as a managed console, Windows Forms or WPF application. It is very flexible option because it requires the least infrastructure to deploy. You need to embed the code inside the managed application to create and open an instance of the ServiceHost to make service available. Services hosted like this are very useful during development because you can easily debug and get trace information to find out what is happening inside the application.
- Windows Service – WCF service can also be hosted as a Windows Service so that it is under control of the Service Control Manager (SCM). Similar to the self-hosting option, some hosting code needed to be written as part of the application. The lifetime of the service is controlled by the operating system which is better option for long running WCF services.
In this tutorial, I tried my best to give you basic introduction of WCF technology, its key features, concepts and architecture. If you are ready to learn more about implementing WCF services or want to learn WCF through code then you can read my other article Programming WCF Services