.NET Delegates are objects which can refer to static and instance methods in memory at runtime and can call those methods in your program. In this tutorial I will show you how you can create and use delegates in C#.
To explain delegates and their use in real world programs I am creating a BankAccount class in which I will declare one delegate using C# delegate keywork.
public class BankAccount
{
int amount = 0;
// Declare Delegate Type Object
public delegate void BankDelegate(int oldBalance, int newBalance);
// Create Delegate Type Events
public event BankDelegate OnDeposit;
public event BankDelegate OnWithdraw;
public void Deposit(int a)
{
// Fire OnDeposit Event and pass old and new balance amount
OnDeposit(this.amount, this.amount + a);
this.amount += a;
}
public void Withdraw(int a)
{
// Fire OnWithdraw Event and pass old and new balance amount
OnWithdraw(this.amount, this.amount - a);
this.amount -= a;
}
}
To test BankAccount class create one Windows Form Application using C# and in the Form Load event create object of BankAccount class as shown below
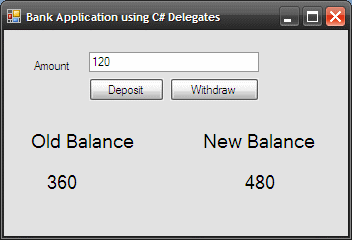
// Declare BankAccount class variable
BankAccount account = null;
private void Form1_Load(object sender, EventArgs e)
{
account = new BankAccount();
// Attach Event Handlers with Events
account.OnDeposit += new BankAccount.BankDelegate(account_OnDeposit);
account.OnWithdraw += new BankAccount.BankDelegate(account_OnWithdraw);
}
Following code is calling BankAccount class methods on Deposit and Withdraw button click events.
private void btnDeposit_Click(object sender, EventArgs e)
{
account.Deposit(Int32.Parse(textBox1.Text));
}
private void btnWithdraw_Click(object sender, EventArgs e)
{
account.Withdraw(Int32.Parse(textBox1.Text));
}
Following methods are event handlers methods attached with delegate type events OnDeposit and OnWithdraw
void account_OnDeposit(int oldBalance, int newBalance)
{
label4.Text = oldBalance.ToString();
label5.Text = newBalance.ToString();
}
void account_OnWithdraw(int oldBalance, int newBalance)
{
label4.Text = oldBalance.ToString();
label5.Text = newBalance.ToString();
}
Nice tutorial. but i feel difficulty in following code:
account.OnDeposit += new BankAccount.BankDelegate(account_OnDeposit);
Please kindly explain it for me.
Waqas,
This is the first piece of Visual C# code I’ve seen dealing with delegates that actually makes sense. Much, much thanks.
My experience with reference manuals is that they are totally divorced from real life programming. So again, much, much thanks.
Still, I don’t have a clear picture of the advantages and disadvantages of using delgates, so any additional information or code on delegates would be helpful.
Ted
.
Sir thank you very much for you post… Its really helpful…
Best delegate tutorial yet…well done
nice tut
very nice
Thanks for give Delegates with Events tutorial,it is helpful for learning dotnet 2.0.
Thanks
Santosh Kumar
http://www.operativesystems…