This tutorial will show you how to use CheckBox inside GridView and how to handle its events to update database records based on its Checked state.
ASP.NET GridView Control provides developers ability to use any type of ASP.NET control in its columns using TemplateField. Developers are free to use Buttons, DropDownList, RadioButtons or any other control according to their application requirement. One of the most common control developers uses in the GridView is CheckBox control and if you are creating Administration Panel of any ASP.NET Application you may be required to handle checkbox event to update any back end database table. One Typical example is to Enable/Disable status of any record in the database table using the CheckBox in the GridView. In the following tutorial I will show you how you can use CheckBox in the GridView which not only display the current status of the record but also update the record status in the database.
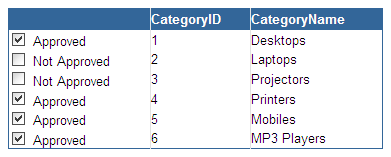
To start this tutorial, I am assuming that you have table in your database with one Bit type column such as Approved in the following figure.
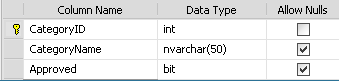
I will update the status of this column to True or False according to the state of the checkbox in the GridView. The Page Load event binds your Database Categories Table to your GridView Control.
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
LoadData();
}
}
private void LoadData()
{
string constr = @"Server=.\SQLEXPRESS;Database=TestDB;uid=waqas;pwd=sql;";
string query = @"SELECT CategoryID, CategoryName, Approved FROM Categories";
SqlDataAdapter da = new SqlDataAdapter(query, constr);
DataTable table = new DataTable();
da.Fill(table);
GridView1.DataSource = table;
GridView1.DataBind();
}
Please keep in mind that I put connection string directly in the code just for the demonstration. You should store your database connection string in web.config file. You can also call stored procedure in the above code if you don’t want to write SQL Query directly in your code or you can also call your Data Access Layer component to retrieve Categories Table from database. The following ASP.NET source will show you how you can use TemplateField in the GridView to display CheckBox with the current status of the Category in the database.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
BackColor="White" BorderColor="#336699" BorderStyle="Solid" BorderWidth="1px"
CellPadding="0" CellSpacing="0" DataKeyNames="CategoryID" Font-Size="10"
Font-Names="Arial" GridLines="Vertical" Width="40%">
<Columns>
<asp:TemplateField>
<ItemTemplate>
<asp:CheckBox ID="chkStatus" runat="server"
AutoPostBack="true" OnCheckedChanged="chkStatus_OnCheckedChanged"
Checked='<%# Convert.ToBoolean(Eval("Approved")) %>'
Text='<%# Eval("Approved").ToString().Equals("True") ? " Approved " : " Not Approved " %>' />
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="CategoryID" HeaderText="CategoryID" />
<asp:BoundField DataField="CategoryName" HeaderText="CategoryName" />
</Columns>
<HeaderStyle BackColor="#336699" ForeColor="White" Height="20" />
</asp:GridView>
In the above code see how I am setting Checked State of the CheckBox from the Approved Column value in the database. I am also setting the Text Property of the CheckBox Approved or Not Approved according to the state of Approved Column in database. To provide user option to update the status of any Category from the CheckBox I am also setting AutoPostBack Property to true and adding Event Handler chkStatus_OnCheckedChanged to handle OnCheckedChanged event. Every time user will check or uncheck the CheckBox this event will update the status of Approved Column in the Database.
public void chkStatus_OnCheckedChanged(object sender, EventArgs e)
{
CheckBox chkStatus = (CheckBox)sender;
GridViewRow row = (GridViewRow)chkStatus.NamingContainer;
string cid = row.Cells[1].Text;
bool status = chkStatus.Checked;
string constr = @"Server=.\SQLEXPRESS;Database=TestDB;uid=waqas;pwd=sql;";
string query = "UPDATE Categories SET Approved = @Approved WHERE CategoryID = @CategoryID";
SqlConnection con = new SqlConnection(constr);
SqlCommand com = new SqlCommand(query, con);
com.Parameters.Add("@Approved", SqlDbType.Bit).Value = status;
com.Parameters.Add("@CategoryID", SqlDbType.Int).Value = cid;
con.Open();
com.ExecuteNonQuery();
con.Close();
LoadData();
}
In the above code, first four lines are doing the main job. I am getting the reference of CheckBox by type casting the sender parameter of the Event Handler. To get the CategoryID I am getting reference of the entire GridViewRow object. The remaining code is straight forward ADO.NET code to execute the Update Query in Database using SqlConnection and SqlCommand object.
Thanks
plz tell code
if we click the checkbox in grid view that should save as true and defautly should save as false in database plz tell the code humble request
awsome solution helped alot
i download it and its awesome effort but i tried many time to connect to my database and it shows a error… any help??
hi, In my case i have to disable only specific rows in the gridveiw which was retrieved fro m the database.So how to disable only specific coloumns and disable the entire row also
Hello sir
thanks for this tutorial but one brolem in this that is woks when checked box is selected but if we click on unselected it remain same no changes
so if any code to solve this problem pls send me on my email id
when approved then how will notify to user
Hi,
this tutorial is really good. I have tried your code for checkbox in grid view.
i have class and property field . when i click on check box . it does not checked .can you help me ?
thanks
This worked really well and inside an updatepanel it is pretty slick! Thanks for posting.
Thanks, this topic is really very helpful
This is the best… helped me a lot. Thank you sooooo much.
hey this is not working for me.
I need to include check box in my grid view.
please help me.
I have a check box in a gridview. I want the user to check multiple and copy those rows to a different table.
hi i am trying in gridview have chekboxes and gridview outside one dropdownlst and button my requirment is when i click button selected checkboxes tasks updated to selected dropdown list mebers cani get code for this
thanks
WOoOw you are my hero…
in others blogs i see this but you are great Teacher and master.
very good explaining.
i say a little pray for you.
jajajaja
thnks!!!
great. clear and concise
Fix :
Input string was not in a correct format.
???
Thank you so much. This article helps me a lot.
I use this code to solve problem but it show error like invalid column name emp_id at categoryID in your code.emp_id is true and valid .Now what i have to do?
That great. I never seen so easy on grid view topic.
It is so help full
Thanx for this.
what a great tutorial sir….
Thank You very much…
Very useful. Thank You.
relay useful thank you so much 🙂
thank.
Thanks a lot for posting this.
It saved my time.
Simple and clear explanation
excellant post
Thank You. This code is very helpfull for me Thanks again
awesome
Why not use Jquery to select all checkbox at client side ?
very easily and simply explained code , helped me a lot , thanx a lot
Thank you for explaining this in such a simple way. Other articles overcomplicated this.
nice code… realy thanx…
Thanks for sharing that!!
I struggeled for hours trying to solve this problem. Your solution (converted to VB) was the cure for my pain.
I am glad that my tutorial helped you in solving your problem
Its Working…
if (!Page.IsPostBack)
{
LoadData();
}
If u miss this part it will not work
Rawler: U r ri8.. it does not fire “OnCheckedChanged” event…
thanks………
I tried this, but still every single click on checkbox control, isn’t firing anything even with autpostback in true; also I dont have any validators or required controls in this form, what could be happenning? VS 2005
Asalam-o-Alaikum
sir can elaborate this sgment while Eval checkbox text value
Eval(“Approved”).ToString().Equals(“True”) ? ” Approved ” : ” Not Approved “
Great! I was trying to have a checkbox be either enable or disabled based on a boolean value in my sql query and this allowed me to do that. Thanks!