When you display records using ASP.NET GridView control it displays all the columns on the page and often you want to display only few columns in GridView due to the available space on the page. You can easily achieve this functionality by adding your own columns declaratively in the GridView control. But if you want to display other columns in a popup window for each row in the GridView control you need to use AJAX Popup Control with the GridView. In this tutorial I will show you how you can display GridView row details using ASP.NET AJAX Popup control extender.
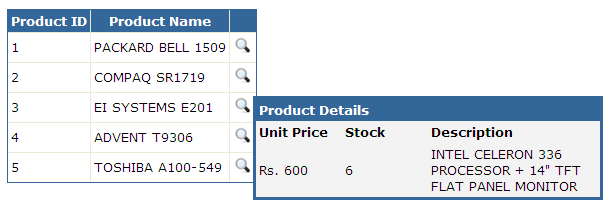
To start this tutorial, create a new website in Visual Studio 2008 and add ScriptManager control on the page. This control is required to use any AJAX functionality on ASP.NET page.
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
Next you need to add GridView control on the page that has AutoGenerateColumns property set to false to stop ASP.NET to generate columns automatically. You also need to attach OnRowCreated event handler with the grid which I will use later in this tutorial to attach some JavaScript events with a magnify icon to display popup window on mouse over. Following is the complete markup of the GridView control.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
BorderColor="#336699" BorderStyle="Solid" BorderWidth="1px"
CellPadding="3" Font-Names="Verdana" Font-Size="10pt"
onrowcreated="GridView1_RowCreated">
<Columns>
<asp:BoundField DataField="ProductID" HeaderText="Product ID" />
<asp:BoundField DataField="ProductName" HeaderText="Product Name" />
<asp:TemplateField ItemStyle-Width="40" ItemStyle-HorizontalAlign="Right">
<ItemTemplate>
<asp:Image ID="Image1" runat="server" ImageUrl="~/images/magnify.gif" />
<ajax:PopupControlExtender ID="PopupControlExtender1" runat="server"
PopupControlID="Panel1"
TargetControlID="Image1"
DynamicContextKey='<%# Eval("ProductID") %>'
DynamicControlID="Panel1"
DynamicServiceMethod="GetDynamicContent" Position="Bottom">
</ajax:PopupControlExtender>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<HeaderStyle BackColor="#336699" ForeColor="White" />
</asp:GridView>
The most important thing in the above markup is the TemplateField column definition in which I have used an image control to display magnify icon and ASP.NET AJAX Popup Control Extender.
The PopupControlID property needs the id of the control you want to use as a popup window with AJAX Popup Control in this case I have used Panel control.
The TargetControlID property needs the id of the control that will display the popup window in this case I have used Image1 control that shows magnify icon in the GridView column.
The DynamicServiceMethod property needs the name of the web service method you want to call for every popup window to load additional details from the database.
The DynamicContextKey property needs the dynamic value you want to pass to the dynamic web service method. In this example I am passing ProductID to load more details of the product in the popup window dynamically.
In the end you need a Panel control on the page which will be used as a popup window by the PopupControlExtender control.
<asp:Panel ID="Panel1" runat="server"> </asp:Panel>
Now I am moving to the code behind file of the ASP.NET page that has Page Load event to bind data with the GridView control as shown below:
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
LoadData();
}
}
private void LoadData()
{
string constr = "Server=TestServer;Database=SampleDatabase;uid=test;pwd=test;";
string query = "SELECT ProductID, ProductName FROM Products";
SqlDataAdapter da = new SqlDataAdapter(query, constr);
DataTable table = new DataTable();
da.Fill(table);
GridView1.DataSource = table;
GridView1.DataBind();
}
Next you need the OnRowCreated event definition that will perform two operations during the creation of every data row of the GridView control. First it will find the Popup Extender Control using the FindControl method and will add a unique behavior id with each popup control. Secondly it will find Image control in every row and will attach showPopup() and hidePopup() script with the image onmouseover and onmouseout events. This is required to display popup window on mouse over and hide popup window on mouse out automatically.
protected void GridView1_RowCreated(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
PopupControlExtender pce = e.Row.FindControl("PopupControlExtender1") as PopupControlExtender;
string behaviorID = "pce_" + e.Row.RowIndex;
pce.BehaviorID = behaviorID;
Image img = (Image)e.Row.FindControl("Image1");
string OnMouseOverScript = string.Format("$find('{0}').showPopup();", behaviorID);
string OnMouseOutScript = string.Format("$find('{0}').hidePopup();", behaviorID);
img.Attributes.Add("onmouseover", OnMouseOverScript);
img.Attributes.Add("onmouseout", OnMouseOutScript);
}
}
In the end, you need the definition of GetDynamicContent service method that will be called by every ASP.NET AJAX Popup Control Extender to load dynamic data from the database and to display additional details in the popup window. The first few lines in the method below are loading additional columns from the Products table in database. The StringBuilder object is created to build html string for the tabular output in the popup window. You can build any type of html for display such as bullets, paragraphs, links etc.
[System.Web.Services.WebMethodAttribute(),
System.Web.Script.Services.ScriptMethodAttribute()]
public static string GetDynamicContent(string contextKey)
{
string constr = "Server=TestServer;Database=SampleDatabase;uid=test;pwd=test;";
string query = "SELECT UnitPrice, UnitsInStock, Description FROM Products WHERE ProductID = " + contextKey;
SqlDataAdapter da = new SqlDataAdapter(query, constr);
DataTable table = new DataTable();
da.Fill(table);
StringBuilder b = new StringBuilder();
b.Append("<table style='background-color:#f3f3f3; border: #336699 3px solid; ");
b.Append("width:350px; font-size:10pt; font-family:Verdana;' cellspacing='0' cellpadding='3'>");
b.Append("<tr><td colspan='3' style='background-color:#336699; color:white;'>");
b.Append("<b>Product Details</b>"); b.Append("</td></tr>");
b.Append("<tr><td style='width:80px;'><b>Unit Price</b></td>");
b.Append("<td style='width:80px;'><b>Stock</b></td>");
b.Append("<td><b>Description</b></td></tr>");
b.Append("<tr>");
b.Append("<td>$" + table.Rows[0]["UnitPrice"].ToString() + "</td>");
b.Append("<td>" + table.Rows[0]["UnitsInStock"].ToString() + "</td>");
b.Append("<td>" + table.Rows[0]["Description"].ToString() + "</td>");
b.Append("</tr>");
b.Append("</table>");
return b.ToString();
}
If you have implemented everything in this tutorial properly you will see the output similar to the figure shown in the start of this tutorial. Please make sure that you have imported the following namespaces in the code file before you run this project.
using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Data;
using AjaxControlToolkit;
using System.Text;
Also make sure that you have registered the AjaxControlToolkit in your aspx file using the Register directive as shown below:
<%@ Register Assembly="AjaxControlToolkit" Namespace="AjaxControlToolkit" TagPrefix="ajax" %>
Feel free to experiment with this tutorial and customize the output and data according to your own requirements.
In the public static string GetDynamicContent (string contextKey) must write the right “contextKey”, but the other word is wrong. For example: GetDynamicContent (string xxxxx) is a Web Service call failed; 500
Popup not show and hide please send this code
can u plz send the code form view pdf file in popup windows from gridview
Thanks Dear,
It Helps to learn me lots
best wishes to you
Hi,
The extender works perfectly fine, when it loads for the first time but fails miserably after a postback, the panel displays varying results of different records and sometimes the there is no pop up at all :(.
could you please help me out ?.
Thanks in advance
Web service call failed errors can be caused by simple errors like incorrect or not matching HTML tags being part of the StringBuilder object etc. Kindly ensure that the arguments in the table.Rows[0].[XXXXXXX] exactly match your table’s fields. This error costed me a day;
Really good work. Thanks a lot 🙂
Nice post, here i have similar article i know am very late to comment here , but its useful for others i.e Gridview row mouse-over show detail data in floating div with jquery , but instead of mouse-over on button, user can mouse-over on each row and data will get display.
http://codepedia.info/2015/…
I am getting error on mouseover on imagebutton
— Web Service call failed; 500
i need javascript methods showpopup and hide popup
Thanks a lot
Dear Sir, nice examples .lot of thanks .
I am getting an error
Web Service Call Failed:12091
Hi, Your code works great!
I have removed the mouseout code, so the popup will stay visible until click outside, But can you please tell how can I highlight corresponding row if the popup is stays visible, Is that possible???
Works great. One thing I would like to do is be able to control the position of the Panel container.
error of web service call fail:500 is cos you should hve missed out these lines:
[System.Web.Services.WebMethodAttribute(),
System.Web.Script.Services.ScriptMethodAttribute()]
try adding it and rock on
Is there any way to do this without using template field??
good works Sir,
thanks………..
Nice Job Done ……………..But I Want To show grid as dialog box and after selecting a single or multiple rows that data should get inserted on parent page……………
nice code but not working well in vb.net?? why so
hey i am getting error of web service call fail:500
please help me!!!
This example displays only 1st row of in popup table. But what if there are multiple rows in the data table. How to display all of them ?
Thank you, Its working good
Thanks
My pop is not working. If I am taking mouse on cell, its not showing me anything. And what about showpopup() and hidepopup(). whats its code?? Plz help. Its very very urgent.
HI, shall i send u my code?? Its very urgent for me so asking..and am very new to .net and ajax.
Thanks waqas, SAM here.As u said i checked but my brkpoint is not hitting to GetDynamicContent() method. Also if condition on row_created event ie
if (e.Row.RowType == DataControlRowType.DataRow)
{
//code
}
is returning false and getting out of loop.
Hi SAM
You set breakpiont in GetDynamicContent method to check if that breakpoint is hitting or not to figure out what you missed in the tutorial. showPopup and hidePopup are builtin client side methods available for PopupControlExtender.
Its a good site for learning.
http://www.ezzylearning.com…
hi,
Thanks for nice demo. but when Product Details popup opens and when i try to copy data e.g description,this pop up disappears. Please let e know how to resolve this problem.
Thanks in Advance
Getting null error on behaviour id of each popupcontrol extender. Please help me how to resolve this issue
I tried this but i got an error in mouser over ” Webservice call failed :500:
Great article. But can you post the VB code for this?
It will be great.
Nice post this is what I was looking for. thanks
Very good and helpfull tutorials sir.
thanks in advance
Is there showPopup() and hidePopup() javascript missing?
for “Display GridView Row Details using ASP.NET AJAX Popup Control”
I would like to thanks you for this topic.
Is it posiable to use implement this with Visual Studio2005
Regards
Great article! 🙂 Thank you so much 🙂
Hello Sir/Madam
I want to know how to search in grid view using ajax…
And also about nested grid view in asp.net using ajax
Getting the following error
web service error 12030
hi Sam you must have missed something. Check the attribute on top of GetDynamicContent method. May be you missed it.
Very helpful article.
Good Job.
I keep getting the Web Service Call Failed: 500 error. It looks like my contextKey is always null. I even forced it to get a value like this… “DynamicContextKey=”value”… but its still null. Any thoughts or help??
Hi Anwar,
This code workes successfully
Really good explanation
Thanks
Regards
Krunal S Rathod
This is really nice article. I am still confused wheter you are using jquery for showpopup and hidepopup. could you please let me know the code for these two function.
Thanks a lot.
I have seen a lot of people complaining about the Web service call failed:500 . It might be because there might be some errors in the syntax of the web service code. Correct them and it should work.
Thanks for the article. It was very helpful . Implemented successfully!
Keep posting new articles and tutorials.
i hav done al d above.and it shows error on mouseover as “Web Service Call Failed :12030”
I want to use three grid view in a page and each grid view need GetDynamicContent functionality, how to use it.
This code works perfectly. Here are some tips to get this working:
1. Need these using statements
using using System;
using System.Data;
using System.Configuration;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using AjaxControlToolkit;
using System.Text;
2. It works fine in ASP.NET 2.0
Hi,
Nice example. Only issue I am running into is that I am not able to see the popup. Is “showPopup” and “hidePopup” javascript functions that we have to write?
can you send me this sample code?
Thanks
Can you replace that table with detailsview in panel1?
excellent code , Tutorial.
Waqas You are really rock……..
Thanks Fahad for your comments.
I am using this exact method to display extended details of the data row items when the mouse hovers over an image in the row. I am having a weird issue though. My gridview is in an Update panel and after a postback occurs I get varying results in the panel. Sometimes the panel works, sometime I get details that are related to a different record. Any thoughts on what might be causing this?
You can do that exact same thing with 2 lines of Div tags and 4 lines of java.
nice code
i was searching for this
thanks
I got it working now. Thank you.
Hi,
Nice example. Only issue I am running into is that I am not able to see the popup. Is “showPopup” and “hidePopup” javascript functions that we have to write?
can you send me this sample code?
Thanks
Sir i worked on this…but i am getting error as web service call failed:500…I am not getting how to solve this..so please do help me out….
provide some live demos with examples above
afain nice work,sir
thanks